Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial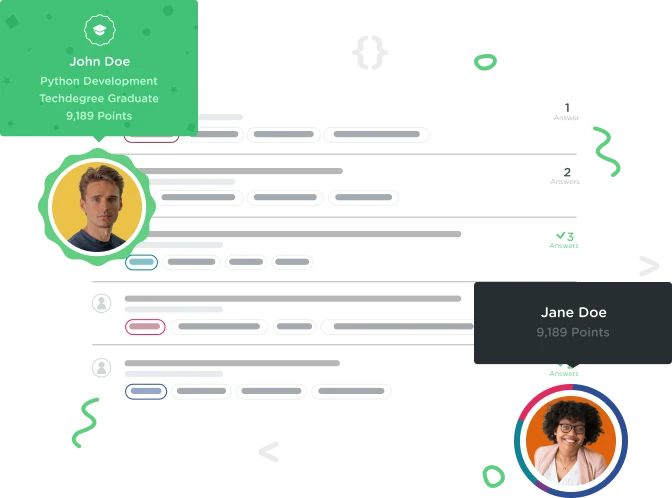
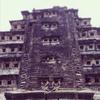
Polo Moreno
11,782 PointsThere are three list items in the index.html file. We want to be able to enter a 0, 1 or 2 in the text field to embolden
There are three list items in the index.html file. We want to be able to enter a 0, 1 or 2 in the text field to embolden the list item with the corresponding index. Can you figure out what condition to put into the if statement to make this code work?
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (law.textContent ) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
8 Answers
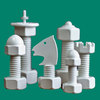
Steven Parker
230,995 Points
Don't over-think this one, it's quite simple.
You already asked this question, but I'll repeat my answer here:
All you need to do here is compare the current loop index with the value that the user has placed in the input box. And to make it easy, the challenge has already created a variable named index and assigned it with the numeric value produced by converting the input field.
You just need to test if the loop index is equal to the user's "index".
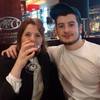
Oliver Sewell
16,425 Points const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
//i is a counter each time the loop runs
let law = laws[i];
// first time the loop runs laws[0] === first list item
// second time the loop runs laws[1] === second list item
//We enter 1 into the input the value is stored in const index.
//first time the loop runs i is 0 does that match our input? no. (fontWeight = 'normal').
//second time the loop runs i is 1 does that match our input? yes! (fontWeight = 'bold').
// third time the loop runs i is 2 does that match our input? no. (fontWeight = 'normal').
if (i == index) {
//update the laws[1] stored in let law to bold
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});

Luis Rabines
20,308 PointsGuil Hernandez hello I am also stuck in this problem can you maybe explain with code the why and how of the answer please. thank you
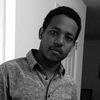
Abinet Kenore
10,082 Pointschange if(false) to if(i == index) // line 12
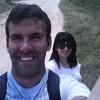
Mihail Mitrevski
Full Stack JavaScript Techdegree Student 8,430 PointsI tried (index=== law) becase law is assignet to the list items index (let law=laws[i])..why is not working?...what is the diffrence between (i===index) and (index===law)?..why this is not the same code?..as i see law and i are quite the same.
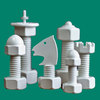
Steven Parker
230,995 PointsNo, "i" represents a number, (0, 1, or 2), but "law" represents an element (one of the list items).

silva celso
13,513 PointsHi steven,
I can not believe i overlooked that. I was looking at the variables at the top and not in the function itself. Totally makes sense. Please dismiss my question.
Thank you for the feedback.

Piotr Manczak
Front End Web Development Techdegree Graduate 29,260 PointsI had this same problem. I think part of it is the fact that we've been given that line: const law = laws[i]; and everyone assumed that it had to be somehow used, hence ( law == index).
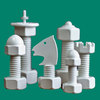
Steven Parker
230,995 PointsThe provided code uses "law" to access the font weight setting, but it's a reference to an element and the comparison is between two integers.

Tomas Rinblad
Full Stack JavaScript Techdegree Student 12,427 PointsAnyone that can explain the 10 number in this code:
const index = parseInt(indexText.value, 10);
What is that one doing there?
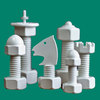
Steven Parker
230,995 PointsIts the conversion radix used for parsing. If you leave it off, 10 (decimal system) is assumed by default.
See the MDN page for parseInt for more information.

brandon may
7,904 PointsI knew the basic concept of what needed to be done but I kept comparing law to index lol.
Luis Rabines
20,308 PointsLuis Rabines
20,308 PointsSteven Parker Can you explain the answer with code please. That would be very helpful thank you
Steven Parker
230,995 PointsSteven Parker
230,995 PointsThe code that would test if the loop index is equal to the user's "index" is:
if (i == index) {
Luis Rabines
20,308 PointsLuis Rabines
20,308 Pointsthank you Steven Parker
Michael Williams
Courses Plus Student 8,059 PointsMichael Williams
Courses Plus Student 8,059 PointsSteven Parker, why must it be:
if (i == index) {
and not:
if (law == index) {
Steven Parker
230,995 PointsSteven Parker
230,995 PointsBoth "i" and "index" are numeric values that can be compared, but "law" is a reference to an HTML element.
silva celso
13,513 Pointssilva celso
13,513 PointsI do not see where you state '''the challenge has already created a variable named index and assigned it with the numeric value produced by converting the input field.'''
where exactly is this? i do not see that anywhere in this challenge. I understand i as being the counter in the loop but what exactly is index? for it is not referenced anywhere in the code?
I was thinking more down the line of what's below to be correct,
if (i == indexText.value) {
Steven Parker
230,995 PointsSteven Parker
230,995 PointsHi silva, it's the first line of the listener code that creates and assigns "index":
const index = parseInt(indexText.value, 10);
It's available for you to use in your comparison expression, as in the example I gave luis and then further explained for Michael.
By itself, "indexText.value" is a string, and should be converted into a number to compare with "i". It's better to do this explicitly than to rely on implicit type coercion. That's why the challenge used "parseInt" to create "index" for you.
Siddharth Pandey
7,280 PointsSiddharth Pandey
7,280 PointsHow come only one of the laws are highlighted, shouldn't all the laws highlight when I put (index == i) into my if statement test condition because the law equals all of the children of the laws which is every list element.