Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial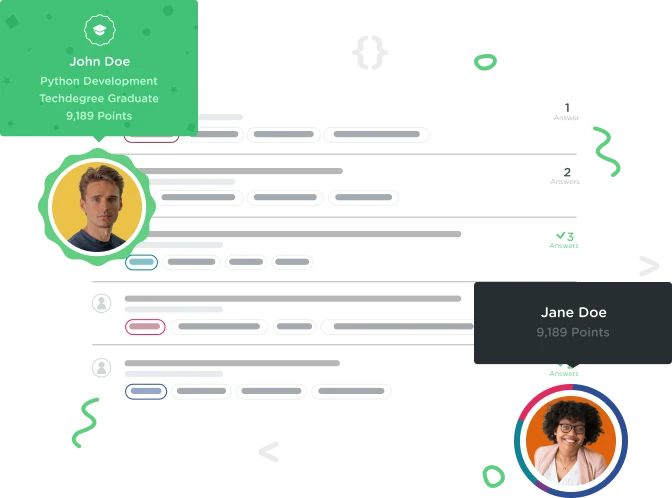

Robert Tardy
142 Pointsthe video is awful, he works in terminal, gives a challenge thats dumb
cant do the problem. i bet a bunch of people cant do this either
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string
String result = "obj";
return result;
}
}
2 Answers

christopher vaughan
1,411 PointsI haven't watched the video as i'm not taking this course, but I think it's asking you to check if the object is a string, and if it is then set result equal to it. so like this:
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
String result = "";
if(obj instanceof String){
result = (String) obj;
}
return result;
}```
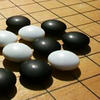
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsYes you do need that typecast. The compiler will not let you reference an Object type with a String variable unless you typecast it into a String first. And typecast will only work if the object is indeed a String, so first you check if the object is an instance of String. It's not "dumb", it's very important. The method takes a parameter of type "Object", that means you can pass anything in it. Could be a String, could be an array of doubles, could be anything. So the method needs to account for that. In fact in the rest of the challenge you will account for another type of object as well. Maybe it will make more sense to you then. If you don't understand it, you better review the videos on inheritance.

christopher vaughan
1,411 PointsOh you're right livia... that's why I added it lol.. it was late and I wasn't thinking lol... so my original code stands OP.
christopher vaughan
1,411 Pointschristopher vaughan
1,411 Pointsyou probably don't need that typecast now that i think of it lol... but it should work regardless. casting a string to a (string) doesn't hurt anything. it just stays the same.