Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial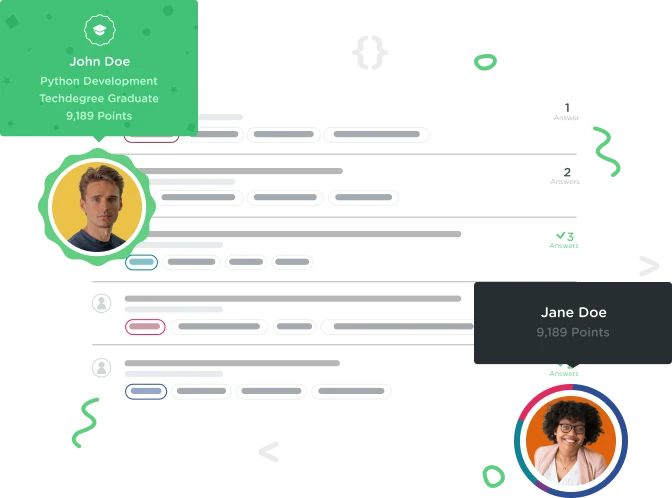

travis halarewich
9,165 PointsThe difference between "players" and "player"
I believe I understand how map() works for the most part as far as creating a new array and iterating through it and applying said map function to every array item. My confusion is coming in with the parameter for the map() function.
const App = (props)=> {
return (
<div className="scoreboard">
<Header
title="Scoreboard"
totalPlayers={props.initialPlayers.length}
/>
{/* Player list starts*/}
{props.initialPlayers.map( player =>
<Player
playerName={player.name}
score={player.score}
/>
)}
"player" is used as this map() function parameter, and is used for "player.name" and "player.score". In normal JS when a function with parameters is called it has to be called with arguments to fill in the code inside the function. Here there is no arguments being passed to the function yet the code runs as if we had passed it the argument of "players" the array with all of the players name and score. My suspicion is it has something to do with that map was called on "initialPlayers". I messed around a bit and changed that parameter form "player" to other things and the code still functions properly. I understand this is the way it is suppose to be done, I am just wanting to understand more of what is actually happening.
1 Answer
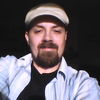
Robert Manolis
Treehouse Guest TeacherHi travis halarewich , Your suspicion is correct. Higher order functions, like map, forEach and filter generally have three things passed to them that you can reference with parameters. Check it out:
arr.map((arrayItem, index, array) => {
console.log(arrayItem);
console.log(index);
console.log(array);
});
Try playing around with that in your code. And like you discovered, it doesn't matter what you name those three parameters. The first will always be the item in the array that's currently being worked on. The next will be the index of the item in the array currently being worked on. And the third will be a reference to the array itself.
One caveat to keep in mind is with the reduce function. That one takes in four parameters, the first being the accumulator, but then the next three will be the same as above.
Hope that helps.
travis halarewich
9,165 Pointstravis halarewich
9,165 PointsThank you Robert for your detailed clarification, you state that the first param for map() will always be the array item that is being worked on. In the code I posted above it only has one param and it seems to me that param is acting like the whole array is being used instead of an array item. I could be wrong on this but I do not have the code in front of me right now to check and mess around with. Again thank you for clarifying after continuing with React and learning about the pre set params for setState() it had helped wrap me head around pre set parameters.