Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial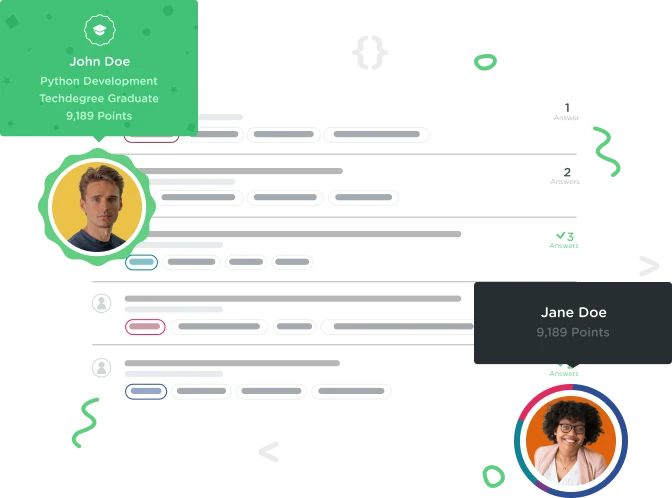

Juan Garcia
10,577 Pointstext content of the a tag to be the value stored in the variable
How can I set the text content of the tag to be a the value stored in the variable linkName?
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
const linkName = document.querySelector('input #link').value('#link');
15 Answers

Joel Kraft
Treehouse Guest TeacherJuan,
Looking at some of your own posted answers, you may have solved this. Just to be clear though (and hopefully to help other students that find this question), let's look at your code here:
const linkName = document.querySelector('input #link').value('#link');
The value you're passing to querySelector
here, 'input #link'
, will return null, because there is only one input on the page, and its id
attribute is linkName
. The error that comes back ('null' is not an object
) is thrown because the interpreter tries to find a value
method on null
. In other words, after resolving document.querySelector('input #link')
to be null
, the interpreter then tries to evaluate null.value('#link')
, and can't do it.
But let's say the input's id, #linkName
, was used, and this was app.js:
const linkName = document.querySelector('#linkName').value('#link');
The issue here is that value
is not a function, so putting parenthesis will result in an error.
There are some points to keep in mind here:
- if you want to select an element by its
id
, use its id only for the css selector, for example'#linkName'
as opposed to'input #linkName'
. This is because there can only ever be one id on the page, so you don't need more specificity. -
<input>
elements have a value property, not a function. To see the value property in use, review this video.
I see there are some answers for the second step here, but you'll need to use the value you got in the first step, and assign it to the textContent
property of the anchor tag.
First you need to select the anchor tag, and you can use its id
they way you did with the input element:
document.querySelector('#link')
Now that it's selected, you can set its text content using the textContent
property, assigning it to the value like so
document.querySelector('#link').textContent = linkName;
Alternatively, you could first store the anchor element in a variable, and then on another line set its text content:
const link = document.querySelector('#link');
link.textContent = linkName;
Hopefully this helps! Click "Add Comment" below if you have more questions.
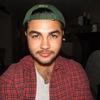
Asai Andrade
Full Stack JavaScript Techdegree Student 2,049 PointsThank you for the thorough and concise explanation to the solution. My issue when trying to solve the problem was the inline syntax. I personally tried using the second option as a solution but always got the error that I needed to go back and revise task 1 since it was no longer passing.
Much appreciated.
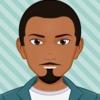
Brian McLaren
17,632 PointsThis worked for me....
let inputValue = document.getElementById('linkName').value;
let link = document.querySelector('a');
link.textContent = inputValue;

Haroon Ghazni
10,093 PointsThanks Brian, yours is one of the only few that worked!

shang jiang
Courses Plus Student 5,830 PointsThis code is correct completely.
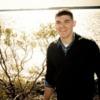
Neil Goldstein
13,671 PointsThanks Brian! Dude, nice save.

Mars Epaecap
5,110 Points//answer format:
//answer to challenge 1
//answer to challenge 2 partA
//answer to challenge 2 partB
// Answer:
let linkName = document.querySelector('#linkName').value;
let randomVariableName = document.querySelector('#link');
randomVariableName.textContent = linkName;
//other formats you can use
let linkName = document.querySelector('input').value;
let anyVariableName = document.querySelector('a');
anyVariableName.textContent = linkName;
let linkName = document.getElementById('linkName').value;
let someVariableName= document.getElementById('link');
someVariableName.textContent = linkName;
let linkName = document.getElementById("linkName").value;
let link = document.querySelector('a');
link.textContent = linkName;
//
// original instructions:
// Challenge Task 1 of 2
//"Get the value of the text input element, and store it in a variable linkName"
//My explanation:
// First, "make a variable named linkName" (they already did this for you on line 1 with,
// let linkName;)
// Step 1B)
//"Get the value of the text input element"
document.querySelector('input').value
// you can also do it this way
// because remember <input>'s id is linkName
document.getElementById('linkName').value
document.querySelector('#linkName').value //# is for id's in case you forgot.
// Step 1C) "store it in a variable linkName"
let linkName = document.querySelector('input').value
// original instructions:
// Challenge Task 2 of 2
// "Set the text content of the a tag to be the value stored in the variable linkName."
//My explanation:
// <a>.textContent = linkName;
// Unfortunately we can't do it like this.
//so we make a random variable set it equal to the a tag <a>
let randomVarName = document.querySelector('a');
//and then set that variable = to link name like this:
randomVarName.textContent = linkName;
//tada! this took me like 2 hours to figure out and another to explain to you.
// ¯\_(ツ)_/¯
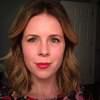
Samantha Hill
Front End Web Development Techdegree Graduate 20,455 Pointsdierkpolzin I had a lot of bugs with this code challenge too! These are the answers they gave me when I emailed the support team: Task 1: let linkName = document.getElementById('linkName').value;
Task 2: let linkName = document.getElementById('linkName').value; document.getElementById('link').textContent = '13f62528e30d754c095240ea3cd2131725528837';
It worked but how? When I asked her to explain how it worked, she said 'Thanks for confirming! Sadly, we in Support are not teachers nor developers, but we're here to aid you with billing, your account and other various matters pertaining to the Treehouse site. We recommend that students use our Community forum to seek out help with their code or further understanding of content in our courses. This would be a great question to ask there. Once you've posted your question on our forum our teachers can then provide assistance. ' I can pass the 1st task in each of these code challenges but the second task always results in an error message. I've been struggling with this issue for 5 days now.

Megan Kleinman
7,008 PointsThank you for posting this. I don't understand why this worked but I have tried every other thing that others have posted to have worked. This section seems terrible and if I didn't need to do it to pass my class, I wouldn't bother. Seems like it was not put together well in the first place and the attempts to rewrite the questions for readability has made things worse. Its a big section but Treehouse needs to admit that this section needs to be scraped and completely overhauled.
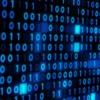
Alexander Davison
65,469 PointsYou can do it like this:
const linkName = document.querySelector('#linkName').value;

Juan Garcia
10,577 PointsI had that answer for the first task. It's the second task that I'm having trouble with. It says to "Set the text content of the a tag to be the value stored in the variable linkName"
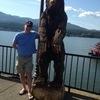
dierkpolzin
7,380 PointsWhat a buggy quiz.... Some of these waste a ton of time.

Jonathan Haro
18,101 PointsYea, I thought it was just me but I don't see as many bugs in other teacher's quizzes
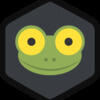
jacknoren
24,134 PointsHere is the answer using "document.querySelector":
let linkName = document.querySelector('#linkName').value; let link = document.querySelector('#content a'); link.textContent = linkName;

mark cromer
7,840 PointsIt's not buggy. Stop blaming the technology and figure out where your understanding is falling short. You're the student here. This code works fine, and is perfectly clear:
var linkName = document.getElementById("linkName").value; const link = document.querySelector('a'); link.textContent = linkName;
What is it that you don't understand? First, you get the value you want to insert, then you find where you want to insert it, then you do the insert.
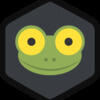
jacknoren
24,134 PointsGood Work!

Mars Epaecap
5,110 Pointslol -10 on this response.
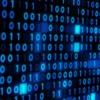
Alexander Davison
65,469 PointsNow -15 votes... Lol...
I once saw a post with 20+ upvotes and I was impressed (it was clearly an amazing answer) but this post seems to have almost the opposite amount of what I saw before...

Sarah Hampton
10,072 PointsI know you got down voted a lot, but your explanation was the one that actually clicked for me. Thanks!

Nick Wilson
5,060 PointsI had a lot of issues figuring this one out. I did this...
let inputValue = document.querySelector('#linkName').value;
const i = document.querySelector('a').textContent = inputValue;

Juan Garcia
10,577 PointsYeah I was able to figure it out like this: const link = document.querySelector('a'); link.textContent = linkName;

Colin Sygiel
5,249 PointsInteresting, I was doing:
a.textContent = linkName;
Not sure why this wasn't working. I guess an ID has to be selected, not a tag name?
Anyways, thanks Juan :)

Juan Garcia
10,577 PointsYou needed to add the a tag in a constant first before storing the value in the linkName variable
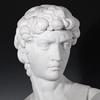
chaz
25,372 PointsHaroon Ghazni the answers posted here are not wrong, they just have a different variable name which Treehouse has since changed in the objective. And as you can see, the created this forum post was looking to store the value in a variable "linkName" not "inputValue". It honestly just sounds like you were copying and pasting without reading the code you were using.

Haroon Ghazni
10,093 PointsSlanderous lies chaz giese, I challenge you to a duel. Though that makes sense why it wasn't working. Cheers.

Anjolaoluwa Akinremi
8,532 PointsThey do not phrase the questions well enough for people to understand.
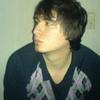
gusf
11,070 PointsSimple explanation :
Challenge : Set the text content of the a tag to be the value stored in the variable inputValue.
Challenge analysed :
- textContent is a property of tag a.
- This property has to have the value stored in inputValue (the value from question 1).
Steps :
- Select the text content of a.
- Give it the value of inputValue.
document.querySelector('#link').textContent = linkName.value;
I hope this helps.
Good luck to you all.

Matthew Conrad
28,069 PointsLike Brian McLaren's solution above, this variation worked just as well.
Challenge Task 1 of 2:
let inputValue = document.querySelector( 'input' ).value;
Declares a variable named inputValue which stores the returned first element within the document that matches the selector input, while the value property gets the value of the attribute.
Challenge Task 2 of 2:
let inputValue = document.querySelector( 'input' ).value;
let a = document.querySelector( 'a' );
a.textContent = inputValue;
Declares a second variable named a which stores the returned first element within the document that matches the selector a, then the textual content of the node--or HTML element--of a is stored in the variable inputValue.
Colin Sygiel
5,249 PointsColin Sygiel
5,249 PointsHey Juan, I am stuck on this one as well. Did you figure it out?