Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial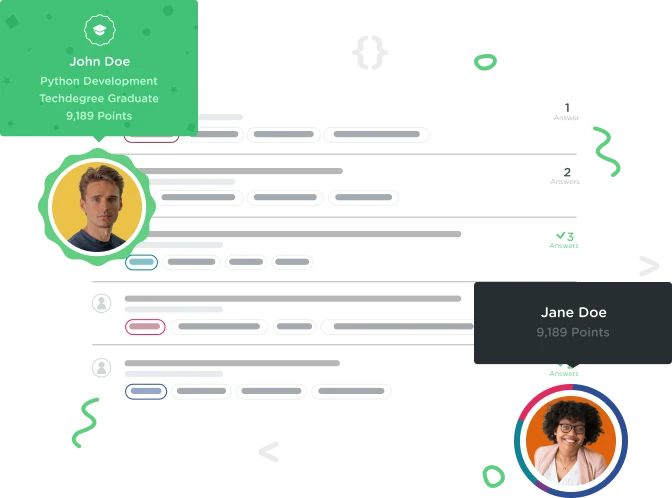

Seth Missiaen
Web Development Techdegree Graduate 21,652 PointsTest possible outputs?
Is it possible to run parts of your code in an environment that will output all possible results? This could be a really convenient way to test more complex math. For example, consider the following code from the random number generator challenge:
// Collect input from a user
let usertop = prompt("Choose a top number");
let userbottom = prompt("Choose a bottom number");
// Convert the input to a number
usertop = parseInt(usertop);
userbottom = parseInt(userbottom);
if (usertop && userbottom) {
// Use Math.random() and the user's number to generate a random number
const userNumber = Math.floor(Math.random() * (usertop - userbottom + 1)) + userbottom;
// Create a message displaying the random number
console.log(`Your random number from ${userbottom} to ${usertop} is ${userNumber}!`);
} else {
console.log('You need to provide two numbers');
}
Is there a way to run the output of this line and see all possible results?
console.log(`Your random number from ${userbottom} to ${usertop} is ${userNumber}!`);
I understand that in most cases this would likely render way too many results, but to test your code you could set the parameters to a small range (e.g. 5 low and 8 high) and hopefully see 5, 6, 7, and 8 as results.
Thanks so much!
1 Answer
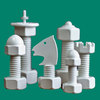
Steven Parker
231,269 PointsThe unpredictability of the random function would make it difficult to test thoroughly without repeating the process a very large number of times and doing some statistical analysis on the output (I've had to do this on occasion!).
But for most purposes it might be sufficient to temporarily replace the random value with the lowest possible value (0) and then run again with the highest possible value (0.999999....) to check that the results fall within the expected range.

Seth Missiaen
Web Development Techdegree Graduate 21,652 PointsThis was quite helpful. I didn't think of simply changing the Math.random()
function to it's highest and lowest parameters to test. Thanks for the advice.
This solution provided by Caleb Kemp did render the result I was looking for:
console.log("The possible results are");
for(let i = userbottom; i <= usertop; i++)
{
console.log(", " + i );
}
Thanks guys!

Caleb Kemp
12,755 PointsGlad to hear it helped
Caleb Kemp
12,755 PointsCaleb Kemp
12,755 PointsPerhaps that could be accomplished with a for loop? Something like
Not sure if that's what you're looking for