Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial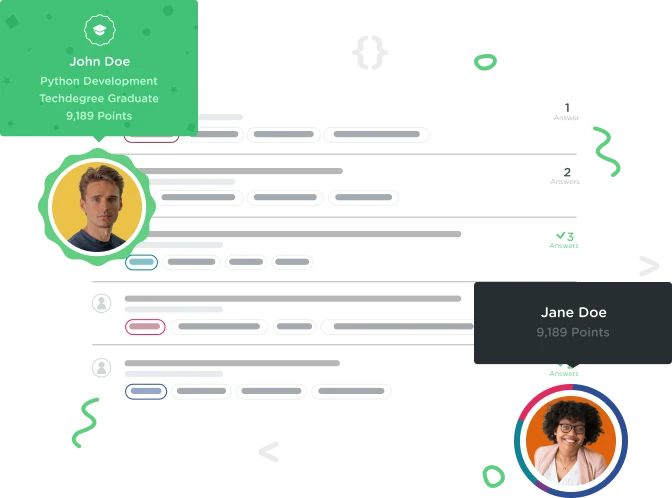

Alana Warson
5,296 Pointstemplate Literal into one string Am I on the right path?
const flavor = "Blueberry"; const type = "Smoothie"; const price = 4.99;
const drink = ` $ {flavor} + ' ' + {type} + ';
const flavor = "Blueberry";
const type = "Smoothie";
const price = 4.99;
const drink = ` $ {flavor} + ' ' + {type} + ': ' + '$' + {price}`;
2 Answers
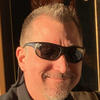
Peter Vann
36,427 PointsHi Alana!
The beauty of template literals is that they save you a lot of typing, so you don't need to concatenate with the + characters.
This passes:
const flavor = "Blueberry";
const type = "Smoothie";
const price = 4.99;
const drink = `${flavor} ${type}: $${price}`;
(Note: you need two dollar signs to the left of "{price}" if you want it to read "$4.99", but, of course only the first one will display - the other is just part of template literal syntax.)
More info:
https://css-tricks.com/template-literals/
https://codeburst.io/javascript-what-are-template-literals-5d08a50ef2e3
I hope that helps.
Stay safe and happy coding!
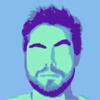
Cameron Childres
11,818 PointsHi Alana,
Some things to keep in mind with template literals -- everything inside of the backticks will appear exactly as you type it, except for variables surrounded by a dollar sign and curly braces. A variable typed like ${this} will be replaced with its value when the template literal is evaluated through string interpolation.
Your current value for drink
will evaluate to this:
$ {flavor} + ' ' + {type} + ': ' + '$' + {price}
The space between the dollar sign and curly braces around flavor
prevents flavor
from being replaced, so it looks exactly like it was typed. Without a dollar sign before ${type}
and ${price}
they won't be replaced either. Plus signs, apostrophes, and spaces should only be included where you want them to appear in the final string.
Hopefully this helps steer you in the right direction! Let me know if you need any further assistance.