Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial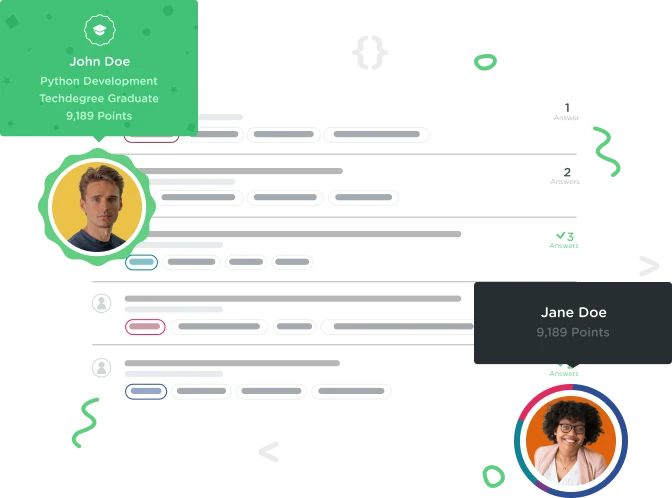
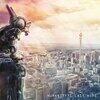
Trenton Spears
Python Development Techdegree Graduate 16,969 PointsTechnical Interview Prep > Basic Python > That's Not My Name
Not sure where I'm going wrong..
Bummer: Uh oh, I didn't get the correct value returned. It should have been False.
def check_name(treehouse, tree):
treehouse = set('treehouse')
{'t', 'r', 'e', 'e', 'h', 'o', 'u', 's', 'e'}
tree = set('tree')
{'t', 'r', 'e', 'e'}
if tree.issubset(treehouse):
return True
if treehouse.issubset(tree):
return False
3 Answers

amandae
15,727 PointsHi, Trenton. I'm sorry the answer didn't work. In my editor it produces one print statement for each if - not "none" I did notice I had a typo in the 2nd "if statement", the second "name1" should be "name2". That typo shouldn't have broken the code - just made the answer slightly confusing.
Here's how I'd answer that question - with notes. I hope this helps!!
# word1 and word2 are parameters - you'll pass the actual words to them when you call the function.
def check_name(word1, word2):
# the next two actions turn the strings into sets.
# these don't print, but you can print them if you want.
# the notation below will print them as a set - not the original string.
word1 = set(word1)
# print(word1)
word2 = set(word2)
# print(word2)
#The question only asks for something to be returned if the 2nd argument is a subset of the first.
#If it isn't true, then nothing happens.
# The question also doesn't ask for the opposite - is the first argument a subset of the second -
# so we don't answer that one, either.
# This answers only what the question asks.
if word2.issubset(word1):
return True
#This is where you put your words.
#make sure to pass them in with '' so that they are strings (and therefore iterable) to start.
print(check_name('treehouse', 'tree'))

amandae
15,727 PointsHi, Trenton. I see a couple of things.
First, the way you have problem set up is a little confusing. Here's the way I would set it up, and I think it is easier to follow. I don't know the question at hand, so if a boolean is needed, this would be set up a little differently, but this will get you started. I find the way the second "if" changes to false is still confusing, but I'm guessing it has to do with the way the question was structured. I would have kept the second "if" just like the first, except change the order of the arguments.
Second, once a function returns, it will not continue. Your returns true, because tree is, in fact, a subset of treehouse. The computer doesn't see any code after it returns. If it idea is having both of them run, then replace the return function with a print function.
def check_name(name1, name2):
name1a = set(name1)
name2a = set(name2)
if name2a.issubset(name1a):
print(f'It is true! {name2} is in {name1}.')
else:
print(f"Nope. Doesn't work. {name2} isn't in {name1}.")
if name1a.issubset(name2a) == False:
print(f"Nope. Doesn't work. {name1} isn't in {name1}.")
else:
print(f'It is true! {name1} is in {name2}.')
print(check_name('treehouse', 'tree'))
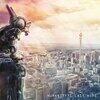
Trenton Spears
Python Development Techdegree Graduate 16,969 PointsHey amandae I appreciate your response! Two differences I noticed between my code and your example:
1) You changed my "set" values from ('treehouse')
and ('tree')
to (treehouse)
and (tree)
.
2) You removed my character list values with the curly brackets for both {'t', 'r', 'e', 'e', 'h', 'o', 'u', 's', 'e'}
and {'t', 'r', 'e', 'e'}
.
The only "Check Work" result difference I noticed was that instead of returning the proper "True" or "False" with those corrections, I received "None".
Do you maybe have another approach or way to explain your process?
Here is the question:
Create a function called check_name. It will receive 2 parameters, both strings. For example: 'treehouse', 'tree'
Turn both strings into sets. Return if the second string ('tree') is a subset of the first ('treehouse'). For example, check_name( 'treehouse', 'tree') should returnTrue`.

amandae
15,727 PointsYou're welcome. Glad to be of help!
Trenton Spears
Python Development Techdegree Graduate 16,969 PointsTrenton Spears
Python Development Techdegree Graduate 16,969 Pointsamandae, I got it! You're awesome thank you so much, I appreciate you!
So echo everything you said but the finishing touch was to top off that cake with an "else" statement to address any other results that weren't in accordance with the answer, like so..