Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial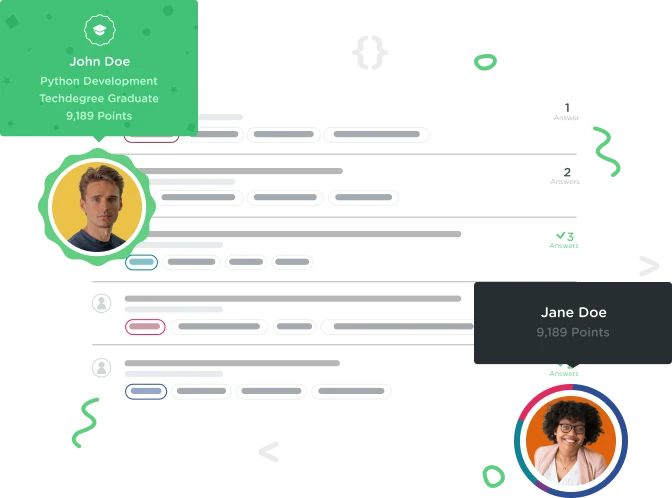
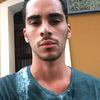
Gregory Millan
3,719 PointsSwitch...What does the switch statement do exactly when entered within a function
Switch...What does the switch statement do exactly when entered within a function
1 Answer
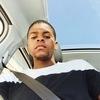
Frezy Mboumba
3,642 PointsHey Gregory, a switch statement allows certain blocks of code to be executed depending on the value of a control expression. Let me show you a simple example on how to use a switch statement within a function. For this particular example, I will make things easy. Let's say I have a function called calculator that takes 2 integers(a & b) and an enum of type Operation.
<p>
enum Operation {
case Addition, Subtraction, Multiplication, Division
}
func calculator(a: Int,b: Int, operation: Operation) -> String {
}
</p>
```
I want this function to return a string containing the result of any operation that I will pass into the function parameter and I want to add, multiply, divide and subtract. However, I don't want to write for every new operation a new function. Swift switch statement comes in handy because it allows us to kill two birds(even more than 2) with one stone. Instead of writing a new function that makes a subtraction for example, I can just switch on the enum value to let the function know what operation I want. Notice something, only the block of operation passed in to the operation parameter will be executed. Down below is how things will all look together:
```html
<p>
// Here is the Operation Enum that will Allow me to switch on operations
enum Operation {
case Addition, Subtraction, Multiplication, Division
}
func calculator(a: Int,b: Int, operation: Operation) -> String {
// This variable returns the result of an operation
var result: String = "No result"
// Notice that after the switch word I used the parameter **operation** because I am switching on the different operations
switch operation {
// Within the switch statement, we can now write the logic for each of the operations
case .Addition:
result = "\(a) plus \(b) is equal to \(a + b)"
return result
case .Subtraction:
result = "\(a) minus \(b) is equal to \(a - b)"
return result
case .Multiplication:
result = "\(a) times \(b) is equal to \(a * b)"
return result
case .Division:
result = "\(a) divided by \(b) is equal to \(a / b)"
return result
}
}
// Now if we call the function calculator and input random numbers such as a = 12 and b = 6 and an operation of our choice, only the block of code corresponding to that operation will be executed and the console will print "12 divided by 6 is equal to 2".
calculator(12, b: 6, operation: .Division)
</p>
```
I hope this example could help you better understand it.