Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial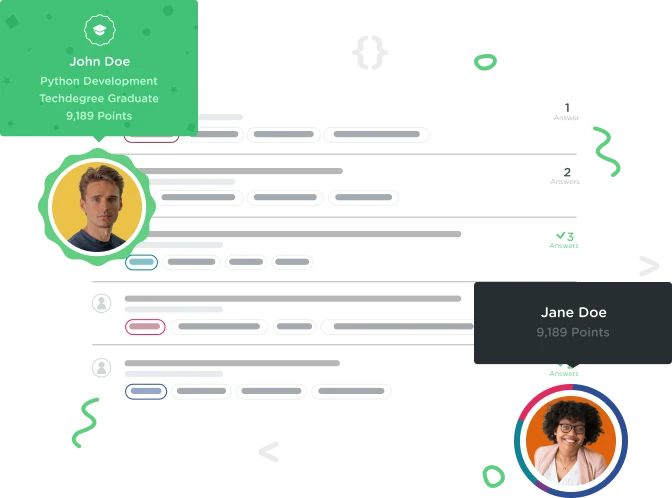

alec apter
929 Pointsswitch statement from playground to Xcode
Hey guys, I want to import this switch function from a lesson into Xcode from a playground:
import GameKit let randomtemperature = GKRandomSource.sharedRandom().nextInt(upperBound: 150)
switch randomtemperature { case 0..<32: print("Its cold") case 32..<45: print("It's cold get a jacket") case 45..<70: print("light sweater") case 70...100: print("wear a shirt") default: print("stay inside") }
Whenever I try to, however, I am met with the error "statements are not allowed at the top level". I know that "top-level code is not allowed in most of your Swift source files, any executable statement not written within a function body, within a class, or otherwise encapsulated is considered top-level" but I can't seem to write it within a function body, class, or encapsulate it to make it work. Any help would be appreciated. Thanks.

alec apter
929 PointsHey Chris, Treehouse won't let me upload a screenshot but I'm using it in the ViewController right under
import UIKit class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
AKA the code that is already provided. Thanks!
2 Answers
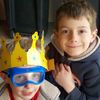
Chris Stromberg
Courses Plus Student 13,389 PointsYour code needs to be placed within the ViewController class. See the example below.
import UIKit
import GameKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
// Your code should go here.
let randomtemperature = GKRandomSource.sharedRandom().nextInt(upperBound: 150)
switch randomtemperature { case 0..<32: print("Its cold")
case 32..<45: print("It's cold get a jacket")
case 45..<70: print("light sweater")
case 70...100: print("wear a shirt")
default: print("stay inside")
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}

alec apter
929 PointsThanks Chris your the man

alec apter
929 PointsAny chance you could help me with creating a button and a label so whenever the button is pushed, a random temperature is created and a case is printed? Iv'e been trying to use other online resources to help but can't get it right. thanks, I know this is a loaded question.
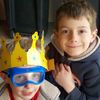
Chris Stromberg
Courses Plus Student 13,389 PointsYou will need to place a UIButton and UILabel onto your storyboard. Once these items are in place you can connect them to your viewcontroller's code where you see @IBOutlet and @IBAction. If your not sure how to do this I would recommend watching "Build a simple iphone app with swift" in the Treehouse Library.
import UIKit
import GameKit
class ViewController: UIViewController {
@IBOutlet weak var yourTextLabel: UILabel!
@IBAction func yourButton(_ sender: Any) {
let randomtemperature = GKRandomSource.sharedRandom().nextInt(upperBound: 150)
switch randomtemperature {
case 0..<32: yourTextLabel.text = "Its cold"
case 32..<45: yourTextLabel.text = "It's cold get a jacket"
case 45..<70: yourTextLabel.text = "light sweater"
case 70...100: yourTextLabel.text = "wear a shirt"
default: yourTextLabel.text = "stay inside"
}
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
yourTextLabel.text = "Press the Button"
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
Chris Stromberg
Courses Plus Student 13,389 PointsChris Stromberg
Courses Plus Student 13,389 PointsCould you paste an example of where you are trying to use it?