Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial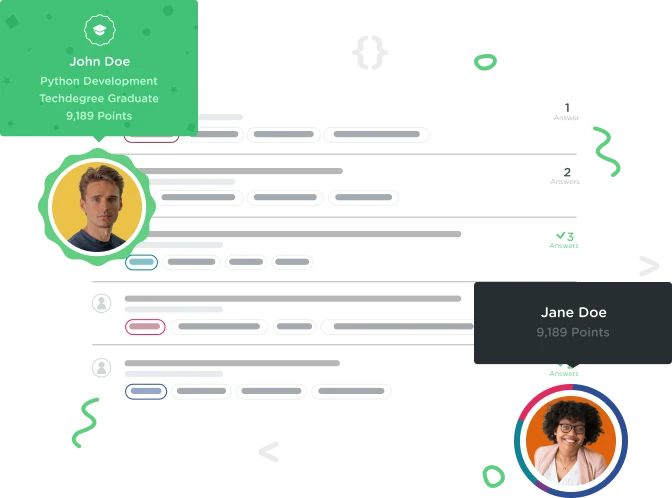

Jolian Chan
4,154 PointsSwift - Use Function to calculate with Dictionary value
Hi,
I'm practicing the function in Swift, I want to make a small calculation with Dictionary as below:
// Input a HKD amount and the currency rate's Key then output the converted result
var rate = ["USD": 0.13, "JPY": 14.40, "SD": 0.17, "SKW": 136.54]
func convert(hkd: Double, rate: [String]) -> Double {
return hkd * rate
}
convert(hkd: 299, rate: "USD")
I think I got something wrong with the type, but I'm not sure how to fix it. Can anyone give me a little hint?
Thanks.
1 Answer

Simon Di Giovanni
8,429 PointsSo, there are a few things wrong with your code
- 'rate' needs to be a String, like this.
func convert(hkd: Double, rate: String) -> Double {
return hkd * rate
}
Now, to access the Double from your dictionary, you input this -> rate["USD"]. This will return an OPTIONAL value.
All value's that come from a dictionary come as an optional. So you are going to need to unwrap it. The best way to do this, is to include the logic INSIDE your function, like this.
func convert(hkd: Double, rateChoice: String) -> Double {
if let unwrappedRate:Double = rate[rateChoice] {
return hkd * unwrappedRate
}
return hkd * 0
}
I have defaulted the function to return '0' if the optional key does not exist. You can try to attempt to write in some error code as a challenge if you'd like!
I have changed 'rate' to 'rateChoice' because the compiler doesn't understand two things named the same
I'm not sure you've learned this yet, so I'll explain the if let statement. Basically, if let code ONLY assigns the value to unwrappedRate if the optional value exists. If the optional does not exist, it does not execute, and it instead skips the if code entirely.
This is a lot of info to take in. If any of this does not make sense please let me know and I can explain again. It's also worth saying there are so many ways to make this happen, this is just a simple one.
The finished working code
var rate = ["USD": 0.13, "JPY": 14.40, "SD": 0.17, "SKW": 136.54]
func convert(hkd: Double, rateChoice: String) -> Double {
if let unwrappedRate:Double = rate[rateChoice] {
return hkd * unwrappedRate
}
return hkd * 0
}
convert(hkd: 299, rateChoice: "USD")