Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial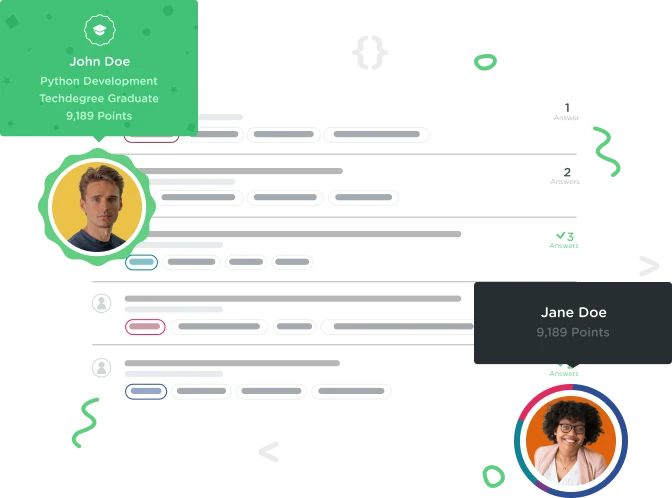
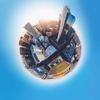
Joel Crouse
4,727 PointsSwift coding practice: how could I improve on this code?
Not too much going on here but would like feedback nonetheless. Could I be doing something better or more efficiently?
// Movie class
class Movie {
let title: String
let genres: [Genre]
// let poster: UIImage
// let discription: String
// let rating: String
// let runtime: String
// let releaseDate: String
init(title: String, genres: [Genre]) {
self.title = title
self.genres = genres
}
func addToGenres() {
for genre in genres {
genre.movies.append(self)
}
}
}
// Genre class
class Genre {
let title: String
var movies: [Movie]
init(title: String, movies: [Movie]) {
self.title = title
self.movies = movies
}
}
// Genres
let drama = Genre(title: "Drama", movies: [])
let thriller = Genre(title: "Thriller", movies: [])
let fantasy = Genre(title: "Fantasy", movies: [])
let scienceFiction = Genre(title: "Science Fiction", movies: [])
func createGenresArray() -> [Genre] {
var genres = [Genre]()
genres.append(drama)
genres.append(thriller)
genres.append(fantasy)
genres.append(scienceFiction)
return genres
}
let genres = createGenresArray()
// Movies
let theShapeOfWater = Movie(title: "The Shape of Water", genres: [drama, thriller])
let wonderWoman = Movie(title: "Wonder Woman", genres: [fantasy, scienceFiction])
let starWarsTheLastJedi = Movie(title: "Star Wars: The Last Jedi", genres: [fantasy, scienceFiction])
theShapeOfWater.addToGenres()
wonderWoman.addToGenres()
starWarsTheLastJedi.addToGenres()
func createMoviesArray() -> [Movie] {
var movies = [Movie]()
movies.append(theShapeOfWater)
movies.append(wonderWoman)
movies.append(starWarsTheLastJedi)
return movies
}
let movies = createMoviesArray()
// Testing
print("Found \(drama.movies.count) drama movies")
print("Found \(thriller.movies.count) thriller movies")
print("Found \(fantasy.movies.count) fantasy movies")
print("Found \(scienceFiction.movies.count) science fiction movies")
print("Total genres: \(genres.count)")
print("Total movies: \(movies.count)")
1 Answer

Cory Waldroup
3,609 PointsAlways consider using a Struct before moving on to using a Class to solve your problems. Using reference types can have pitfalls that value types avoid. Great work though!