Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial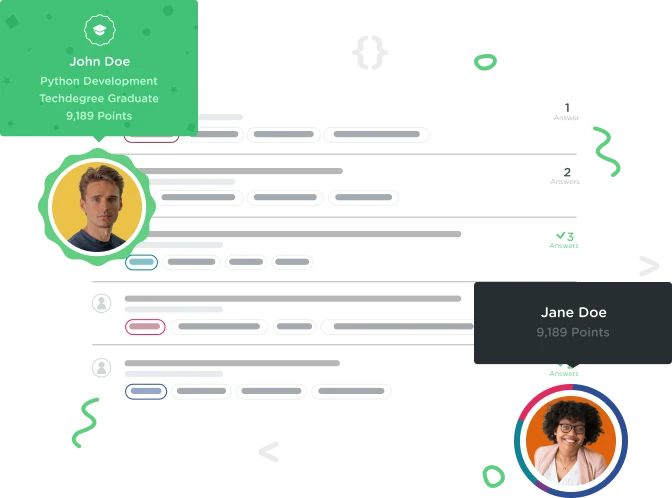
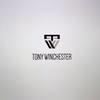
Tony Winchester
11,462 PointsStuck on Swift 3: Working with operators Challenge Part 2
This is the Question:
In this game of ours, we have an odd scoring mechanism . At the end of the round, if your score is 10, you lose! If it's anything but 10, you win.
Declare a constant named isWinner and assign the results of a comparison operation to check whether the player has won or not. For example, if the total score is not 10, then the player has won and isWinner should equal true. (Hint: Use the NOT operator)
What is wrong with my code below? I get an error telling me I should make sure to use the NOT operator.. But obviously I did! Why is the system not accepting my code?
// Enter your code below
var initialScore = 8
let totalScore = initialScore += 1
let isWinner = (totalScore != 10)
3 Answers
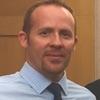
Toby Morgan
iOS Development Techdegree Graduate 23,133 PointsChange the line:
let totalScore = initialScore += 1
With:
let totalScore = initialScore + 1
(i.e. remove the extra equals assignment operator)
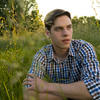
Tomas Salas
Front End Web Development Techdegree Graduate 37,934 Pointslet totalScore = initialScore += 1
you are trying to use Compound Assignment Operator in a circumstance that is not build for it.
try using a unary operator instead ...initalScore + 1
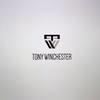
Tony Winchester
11,462 PointsThank you for the suggestions, but if I do that it still doesn't work, I get the response "Make sure you use the unary plus operator to increase the initial score by 1"
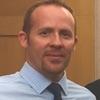
Toby Morgan
iOS Development Techdegree Graduate 23,133 PointsSo if it wants you to incremet using the unary operator do
let totalScore = initialScore++
or
let totalScore = ++initialScore
Depending on whether you want to increment it before or after the assignment.
BTW - the unary operators ++ and -- are deprecated in Swift 3.
Stas Mosin
Courses Plus Student 4,045 PointsStas Mosin
Courses Plus Student 4,045 Pointsvar initialScore = 8
initialScore += 1