Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial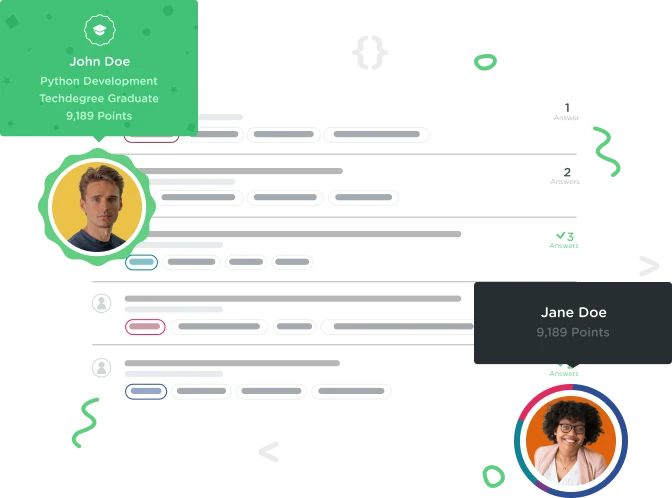

nobodyinhere
3,418 PointsStuck on Sets and Collections Challenge
I can't figure out what exactly we should do in Sets. Also I guess I can't understand the fundamentals of Generics and Collections. I tried many different things but in every time I got some console errors. I understood the example in Video but Still can't do it for BlogPost code series. Maybe I can figure out when I understood what should I do with loops and returning "java.util.Set" of all the authors.
Also We have to sort them alphabetically with TreeSet<String> but when I created new one, I got some critical errors with syntax. What should i do?
Here is task:
I've added a new class called Blog. It is initialized with a list of blog posts. Create a method in the Blog class called getAllAuthors that loops all the posts and returns a java.util.Set of all the authors. They should be sorted alphabetically.
2 Answers

Ken Alger
Treehouse TeacherAbdullah;
Let's have a look.
Challenge
I've added a new class called
Blog
. It is initialized with a list of blog posts. Create a method in theBlog
class calledgetAllAuthors
that loops all the posts and returns ajava.util.Set
of all the authors. They should be sorted alphabetically.
Let me lay out my strategy on this and you can have another go at it with coding it out.
- Create the
getAllAuthors
method... should be a public method that returns aSet
. - Define a working variable. Since it will be what we are returning it needs to be a
Set
. Since it will be a set of authors, that sounds like it needs to be aSet<String>
. Probably need to make sure we importjava.util.Set
. That will be our interface. - Since we are wanting to have the authors returned in alphabetical order, if we implement a
TreeSet
, like you mentioned in your post, we can get that. Probably need to make sure we importjava.util.TreeSet
. - Need to cast our working variable to
TreeSet
, something likeSet<String> authors = new TreeSet<String>()
. - Loop through our posts (
mPosts
) and extract the author from the post and add it to our working set.
- Return our data.
See if that gets you headed in the correct direction and post back if you are still stuck.
Happy coding,
Ken

Yigit Cakar
Courses Plus Student 5,975 PointsHello Abdullah,
Your method should return a set of strings. We are looping through BlogPosts and you forgot to extract the authors.
My getAllAuthors method is as follows:
public Set<String> getAllAuthors(){
Set<String> authors = new TreeSet<String>();
for (BlogPost posts:mPosts){
authors.add(posts.getAuthor());
}
return authors;
}
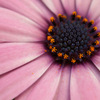
Yael P.
2,816 PointsHello Yigit,
Could you help me understand why your wrote (step by step):
- At the For loop mPosts and not getPosts()
- At the For loop statment ...(posts.getAuthor());
for (BlogPost posts:mPosts){
authors.add(posts.getAuthor());
}
Thanks, Yael

Pedro nieto Sanchez
28,169 PointsHello Yael I think I might help you with this.
1.- Because mPosts has been defined inside the class. (Look at the first line inside the class) So you don't need the getPosts().
2.- Because Posts is each Object inside of the List<BlogPost> mPosts, therefore they all have available the method getaAuthor()
nobodyinhere
3,418 Pointsnobodyinhere
3,418 PointsI guess I can't understand this stage. Where is my fault?
This is my Blog.java