Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial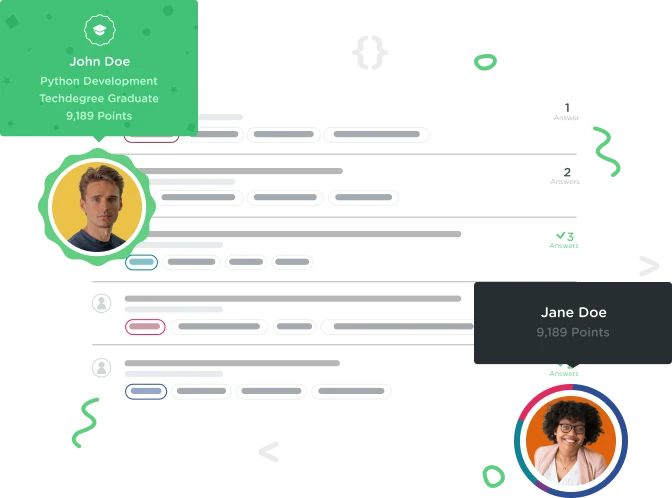

Jeff Quarnberg
Courses Plus Student 11,572 PointsStuck on first_class.py. "Didn't find the name in the praise message. Be sure to use the instance attribute!"
I am still unclear on instances of the class and how I would use the instance in the return. Any guidance would be very appreciated.
class Student:
name = "Your Name"
def praise(self):
return "You're doing a great job {}!".format(self)
Bob = Student()
Bob.praise()
2 Answers

Jon Mirow
9,864 PointsHi there!
You're really close, the issue here is just understanding the idea of the self variable in python.
At the moment your praise method returns something like:
You're doing a great job <__main__.Student object at 0x7f82ce2faeb8>!
Huh?? What's happened here?
When we declare a new class, we're just building a template for instances of that class. Think of it like building a factory that makes .... kitchen appliances (it could be anything - in this example it's a factory that makes students, but that's a bit Matrix...). The factory has all the tools required to build a kitchen appliance and all of the things that go into a kitchen appliance, but it isn't a kitchen appliance - that's what an instance is.
When you do
bob = Student()
Our student factory makes a student and puts that in the variable named bob (by the way you don't have to capitalise the variable that holds the instance of the class, just the class itself)
Now one class could by used to make thousands of instances - all the students in a school for example. Each one has to have it's own attributes like name. We can't use a common name for all students, so we need a way to tell python that when we make a student, the name being applied will be just for that instance of the student. This is where self comes in. Self tells python you're talking about something related to this instance only. The way it does that is that when you type self, you select that specific instance.
So in your code,
<__main__.Student object at 0x7f82ce2faeb8>
Is just how python sees the variable bob internally, or rather it's what bob points to in memory. If we thing about it like that, it's quite easy to use self. If self is just the instance (bob) of the class, then we get at its attributes just like normal:
print(self.name)
Your Name

Jeff Quarnberg
Courses Plus Student 11,572 PointsThank you so much! The factory analogy was very helpful.