Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial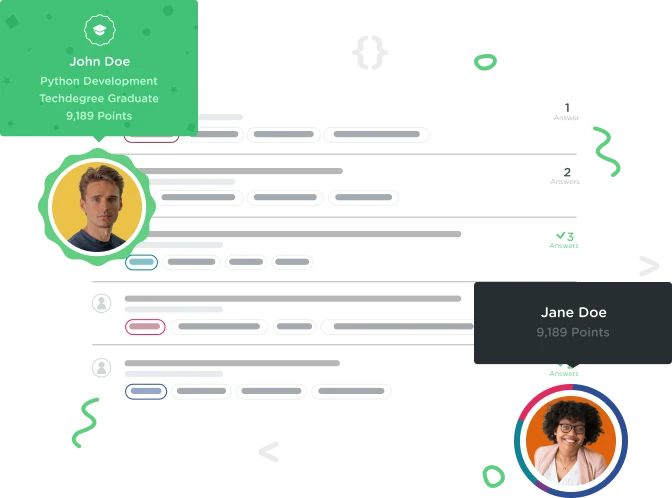
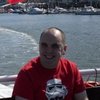
Sean Flanagan
33,236 PointsStuck on BlogPost.java
Hi.
I've been stuck on this for days despite several hints as to how to solve it as I'm thinking maybe I've not got the aptitude. I'd really appreciate some help and explanations as we go.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts) {
if ( counter == null ) {
counter = 0;
}
counter++;
categoryCounts.put(post.getCategory(), counter);
}
return categoryCounts;
}
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
Mod Edit: Changed question title.
5 Answers

Craig Dennis
Treehouse TeacherLooks like you accidentally got rid of:
public Map<String, Integer> getCategoryCounts() {
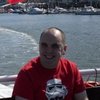
Sean Flanagan
33,236 PointsHi Craig.
So if I'm understanding you correctly, the code should look like this: ?
public Map<String, Integer> categoryCounts = new HashMap<String, Integer>
for (BlogPost post : mPosts) {
String category = post.getCategory();
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count ++;
categoryCounts.put(category, count);
}
return categoryCounts;
Thanks. :-)
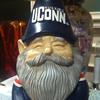
Joshua Briley
Courses Plus Student 24,645 PointsI wish I could help you solve the issue. I don't have any experience with Java. What I can say is "please keep at it, it'll come." If you can't get your questions answered here, I'd recommend heading over to stackoverflow.com. That community is great when it comes to helping each other out. Those folks have bailed me out on numerous occasions. You'll get it, Sean! Just give it time.
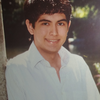
Jon Kussmann
Courses Plus Student 7,254 PointsHi Sean,
It looks like you have not declared your counter variable. Is it a String? BlogPost? int?
I hope that helps.
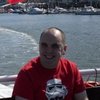
Sean Flanagan
33,236 PointsThank you both for your replies and apologies for my desolate tone.
I'm getting a compiler error. Below are the details:
"./com/example/Blog.java:15: error: cannot find symbol
if ( counter == null ) {
^
symbol: variable counter
location: class Blog
./com/example/Blog.java:16: error: cannot find symbol
counter = 0;
^
symbol: variable counter
location: class Blog
./com/example/Blog.java:18: error: cannot find symbol
counter++;
^
symbol: variable counter
location: class Blog
./com/example/Blog.java:19: error: cannot find symbol
categoryCounts.put(post.getCategory(), counter);
^
symbol: variable counter
location: class Blog
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
4 errors"
Any help would be great. If you can provide explanations with each part I get wrong, telling me where I went wrong and what I should do instead, I'd be most grateful. :-)
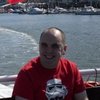
Sean Flanagan
33,236 PointsHi Jon. Thanks for your reply and sorry for the delay; I've had a busy day.
I've worked on it again and here's what I've come up with for Blog:
package com.example;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
Map<String, Integer> categoryCounts = new HashMap<String, Integer>
for (BlogPost post : mPosts) {
String category = post.getCategory();
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count ++;
categoryCounts.put(category, count);
}
return categoryCounts;
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
And the errors:
./com/example/Blog.java:21: error: '(' or '[' expected
for (BlogPost post : mPosts) {
^
./com/example/Blog.java:21: error: illegal start of type
for (BlogPost post : mPosts) {
^
./com/example/Blog.java:21: error: ';' expected
for (BlogPost post : mPosts) {
^
./com/example/Blog.java:21: error: expected
for (BlogPost post : mPosts) {
^
./com/example/Blog.java:21: error: expected
for (BlogPost post : mPosts) {
^
./com/example/Blog.java:30: error: illegal start of type
return categoryCounts;
^
./com/example/Blog.java:30: error: ';' expected
return categoryCounts;
^
./com/example/Blog.java:21: error: cannot find symbol
for (BlogPost post : mPosts) {
^
symbol: class post
location: class Blog
./com/example/Blog.java:21: error: cannot find symbol
for (BlogPost post : mPosts) {
^
symbol: class mPosts
location: class Blog
./com/example/Blog.java:22: error: cannot find symbol
String category = post.getCategory();
^
symbol: variable post
location: class Blog
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
10 errors
Thanks :-)
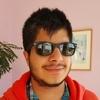
faraz
Courses Plus Student 21,474 PointsHey Sean,
If you're still stuck on this, check out these two links:
https://teamtreehouse.com/community/java-help-7 https://teamtreehouse.com/community/error-again-2
Enrique Munguía
14,311 PointsEnrique Munguía
14,311 Pointswhat is the exact problem with your code?