Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial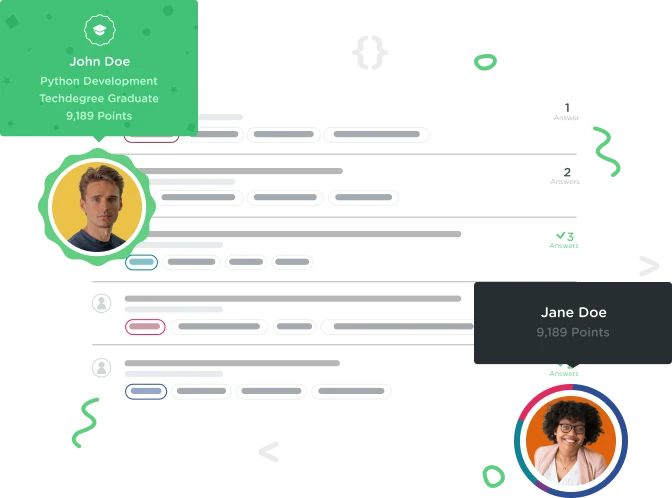

Tatenda Madzokere
2,970 PointsStuck again , i dont know what im doing wrong
var arrayOfInts: [Int] = [1, 2, 3, 4, 5, 6]
im new to swift is there something else i can do to better understand this
// Enter your code below
var arrayOfInts: [Int] = "1,2,3,4,5,6"
1 Answer
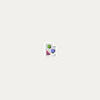
doesitmatter
12,885 PointsOk so this challenge has 4 parts, in each part you are asked to do:
1. make a list. In swift to create a list you always start with brackets []
you put all the values you want in this list, seperated by a comma, like so:
let myList = [1, 2, 3, 4]
2. add an item to the array you created in step (1) in two different ways. In swift to add an item to an array two ways are:
using .append
, or the +
operator which is the most intuitive.
myList.append(5)
//myList now contains: [1, 2, 3, 4, 5]
myList += [6]
//myList now contains: [1, 2, 3, 4, 5, 6]
//NOTE: that a += b is short for: a = a + b
3. retrieve a value from the array you created in step (1) and store it in a constant named value
. To retrieve a value from a list or array in swift, all you need to do is write the name of the list and brackets []
with the index of the value you want to retrieve. NOTE that the index of the first item is 0 the second is 1 and the third is 2 etc.
let value = myList[0]
//value now contains 1
let value2 = myList[4]
//value2 now contains 5
4. remove a value from the array you created in step (1) and store it in a constant named discardedValue
. Finally, to remove an element from swift, we use a function called remove(at: )
. We call it on a list using a dot and pass it the index of the item we want to remove, the function then returns the value we removed from the list.
let discardedValue = myList.remove(at: 0)
//The list now contains: [2, 3, 4, 5, 6]
//discardedValue now contains 1
let discardedValue2 = myList.remove(at: 3)
//The list now contains: [2, 3, 4, 6]
//discardedValue2 now contains 5