Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial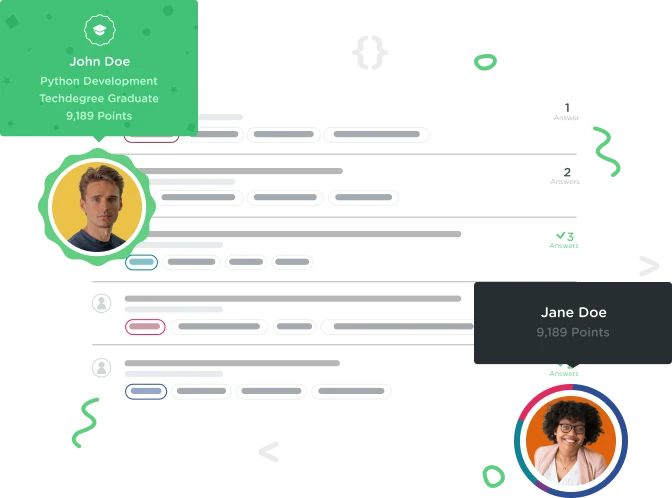
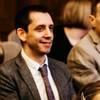
Leon Segal
14,754 PointsStrange if else problem
Hi
I am stumped; I have written out the code which works and does this:
- searches for a student
- if the student is found, prints the student's record
When I tried to add an extra part to the code to display a message to let the user know the student wasn't on the list, something strange happened!
It's line 41 below:
1 (function () { //js lint demanded this
2
3 'use strict'; //and this
4
5 var message = '', //to hold string of html that will build up in a loop and print out to the document
6 student, //holds student object each time the loop runs
7 outputDiv, //the div where the code will display
8 i, //for the loop counter
9 search; //what the user will enter to search
10
11 //to output the strings needed
12 function print(message) {
13 outputDiv = document.getElementById('output');
14 outputDiv.innerHTML = message;
15 }
16
17 //to get the strings needed if the search produces a result
18 function printStudent(student) {
19 student = students[i]; // set student variable for ease of reading
20 message = '<h2>Student: ' + student.name + '</h2>';
21 message += '<p>Track: ' + student.track + '</p>';
22 message += '<p>Achievements: ' + student.achievements + '</p>';
23 message += '<p>Points: ' + student.points + '</p>';
24 print(message);
25 }
26
27 document.write(students[0].name); //testing to see if the program could read from the array
28
29 while (true) { //endless loop
30 search = prompt("Enter the name of the student (quit to exit)"); //get name of student
31 // console.log(search + typeof search); //just a test to see if the search matched the array
32 // console.log(students[0].name + typeof students[0].name); //another test
33 if (search.toLowerCase() === 'quit' || search === null) { //break points
34 break;
35 } else {
36 for (i = 0; i < students.length; i += 1) { //loop through students names
37 student = students[i]; // set student variable for ease of reading
38 if (search === student.name) { //check student name
39 printStudent(search); //print student name if in array
40 } else if (search !== student.name) {
41 print("No such student"); //this is weird; if commented out, the program works, but if left in it deafults the 42 output to "No such student"
43 }//end if
44 } //end for
45 } //end else/if
46 } //end while
47
48 //To do: use push method to add students with same name to an array then print that array
49
50 }()); //end anonymous function
If I comment the line out, the program works fine, but if it's left in, the search displays this, no matter what the user enters as the search variable!
Any help gratefully appreciated!!
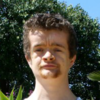
Peter Smith
12,347 Pointswithout running your code, my hypothesis is that you're running a search and finding a match, then the loop continues and doesn't find a match, triggering the else if
logic.
I'd check my hypothesis by adding a log console.log
inside the if
and else if
blocks to see if the if
is being triggered before the else if
3 Answers
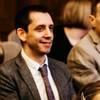
Leon Segal
14,754 PointsPeter Smith 10,718 13m ago without running your code, my hypothesis is that you're running a search and finding a match, then the loop continues and doesn't find a match, triggering the else if logic. I'd check my hypothesis by adding a log console.log inside the if and else if blocks to see if the if is being triggered before the else if
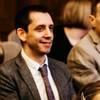
Leon Segal
14,754 PointsSolved - thanks Peter! I added a break after the successful if and it now works - deep joy! :)
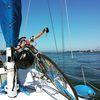
Keenan Wells
2,164 PointsI had the same problem, can anyone explain why this happens and the break is needed?
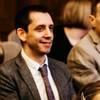
Leon Segal
14,754 Pointsboth statements are executed without the break:
even if the if test is true, the else logic is still tested and then the else statement is run (as it was last thing run).
see what happens if you search for the last student in the list's name, before you add the break! ;)

Patrick Mead
7,487 PointsI had the exact same problem and the break worked for me as well. I understand that the code is running checking the condition of both the if and else statements, but if the condition for the else statement is: (search !== student.name) shouldn't the else statement still come up false and not run, defaulting to the results from the if statement?
birdman
9,450 Pointsbirdman
9,450 PointsI have to leave but something I have noticed is quite a bit of code is mixed up(some parts are put before others when they shouldn't be) I suggest you go back over the video and look at it, I'll check back in later and do a more extensive search though.