Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial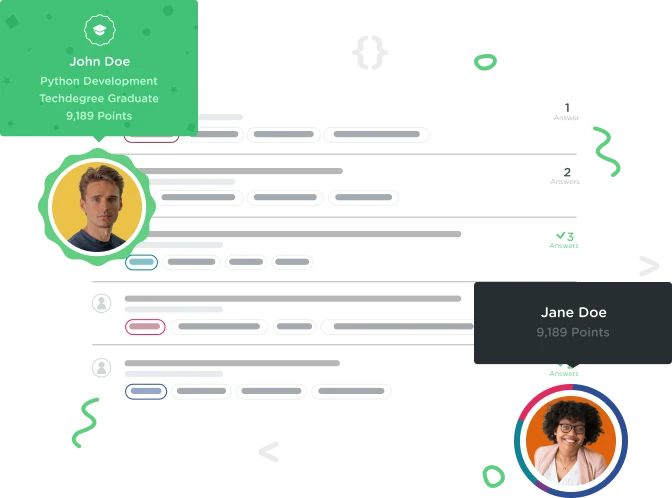

Andrew Phythian
19,747 PointsStarting a new game
I've been playing around with the code a bit and added a counter for keeping score of the number of wins each player has. Now I want to add a 'New Game' button the appears after the current game has finished. I want to achieve it without refreshing the page so that those win/loss counts are preserved.
I initially figured it could be done easily by running a method to empty all of the accumulated player and token data that is generated during a game and starting over. However, given that this is object based the process should surely be easier by creating a new variable for a new instance of the Game class.
Something like
const game2 = new Game();
and then updating the code below in app.js to replace 'game' with 'game2'.
start.addEventListener('click', function () {
game2.startGame();
this.style.display = 'none';
playArea.style.opacity = '1';
});
document.addEventListener('keydown', function(event) {
game2.handleKeyDown(event);
});
It's proving to be tougher than it really should be.
The html 'New Game' button has to initiate the process of updating the variable and the corresponding code. Maybe it could be hard coded into an array beforehand? Or dynamically generate a new button each time (like button 2 corresponds to game2, incrementing it by 1 each time a game ends)?
Any ideas would be welcome.
2 Answers
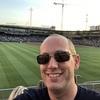
Ian Ostrom
Full Stack JavaScript Techdegree Student 10,332 PointsAndrew Phythian, I was chasing runaway snowballs trying to do something similar. Someone posted a simple solution in another thread.
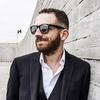
Anwar Montasir
Treehouse TeacherI was experimenting with this question a bit and I think this solution works.
Step 1: make the restart button appear in the DOM when game ends, as part of gameOver
function
gameOver(message) {
const gameOver = document.getElementById('game-over');
gameOver.style.display = 'block';
gameOver.textContent = message;
/* enable restart */
document.getElementById('begin-game').style.display = 'block';
}
Step 2: rework how app.js works a bit so beginning the game works even if it's a second game. My tasks as I saw them to restart the game were
- remove any tokens from the DOM
- hide the 'game over' message once the new game starts
- move the
new Game()
declaration inside the click listener, so a new Game gets generated each time begin game is clicked. This one is critical, otherwise you need to reset a bunch of game settings manually.
const beginGame = document.getElementById('begin-game');
beginGame.addEventListener('click', e => {
/* if tokens are in DOM, clear them */
clearTokens();
/* if game over message is showing, clear it */
document.getElementById('game-over').style.display = 'none';
/* start new game! */
const game = new Game();
e.target.style.display = 'none';
document.getElementById('play-area').style.opacity = '1';
game.startGame();
game.handleKeyDown();
})
function clearTokens() {
const gameBoard = document.getElementById('game-board-underlay');
const gameBoardChildren = gameBoard.children;
/* remove every child but the first */
while (gameBoardChildren.length > 1) {
gameBoard.removeChild(gameBoard.lastChild);
}
}
You'll notice my comment remove every child but the first
. The game board underlay ID has one div inside it initially, I'm leaving that one there and deleting any subsequent children.
I've tested a few games in a row and it seems to work ok. https://github.com/anwarmontasir/four-in-a-row