Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial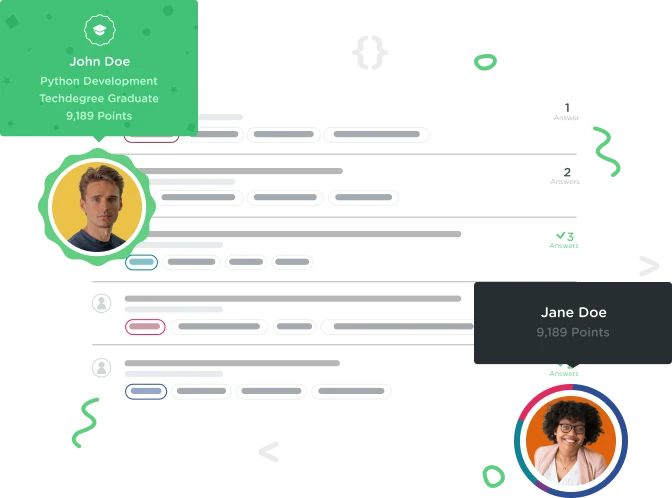
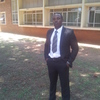
Brett Dube
2,653 Pointsstage 3 challenge i of 2
your code took too long to run, what does this mean?
/* So the age old knock knock joke goes like this:
Person A: Knock Knock.
Person B: Who's there?
Person A: Banana
Person B: Banana who?
...as long as Person A has answered Banana the above repeats endlessly
...assuming the person answers Orange we'd see
Person B: Orange who?
...and then the punchline.
Person A: Orange you glad I didn't say Banana again?
(It's a really bad joke that makes it sound like "Aren't you glad I didn't say Banana again?")
Let's just assume the only two words passed in from the console from Person B are either banana or orange.
*/
// ====BEGIN PROMPTING CODE====
String Who;
Who = "Banana";
do {
// Person A asks:
console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who:
String who = console.readLine("Who's there? ");
// Person B responds:
console.printf("%s who?\n", who);
}
// ==== END PROMPTING CODE ====
while (Who.equalsIgnoreCase ("Banana") );
4 Answers

Ken Alger
Treehouse TeacherBrett;
In the instance of the code posted above, the error is referencing the fact that your do...while loop will never end.
- You have two different variables named
Who
andwho
, remember that Java is case sensitive. - You have assigned your
Who
variable with the value of "banana" and are having the code run the do portion whileWho
is equal to "banana". Since it never changes, or is given the option to change, it winds up in an endless cycle. - You are defining your String object
who
inside the do...while section. That will cause errors as well as it doesn't need to be assigned a type each time through the loop.
Ultimately you will want it to look similar to:
String who;
do {
// something magical
who = console.readLine("Who's there? ");
// more magical code
} while (who.equalsIgnoreCase("someWordToCheck");
Since you are very close to the solution, just some minor syntax adjustments, I will let you proceed from there so that you still have the opportunity to learn and not just give you the answer.
Post back, however, if you are still stuck.
Happy coding and welcome to Treehouse!
Ken
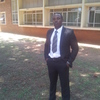
Brett Dube
2,653 PointsThank you so much Ken but i am stil stuck on this challenge, my head is going crazy right now
its now saying " variable who is already defined"

Ken Alger
Treehouse TeacherBrett;
I understand. Without seeing your updated code it is difficult to diagnose the issue. If you utilize the code I posted previously, add the necessary code snippet outputs, and make the changes for the while loop check value, you should be good.
The error you were getting in your last post would lead me to believe that you are declaring the variable who
in more than one place.
If you are still stuck, post your latest code and we'll see where the issue is located.
Thanks,
Ken

Tyler Anyan
2,976 PointsBrett - I was having similar issues, made harder for me because for some reason the compiler errors don't show in the browser I'm using but the solution was super simple; make sure to remove "String" from the who variable when it's called inside the do portion of the loop, ie:
do {
// Person A asks:
console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who:
who = console.readLine("Who's there? ");
// Person B responds:
console.printf("%s who?\n", who);
}
This may or may not be the issue you're having but I know it solved it for me.
(sorry, don't use markdown often enough and can't seem to get it to proper code highlighting)