Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial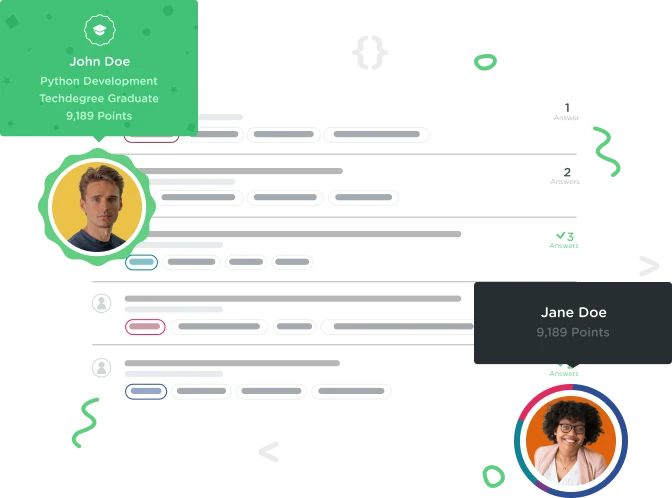
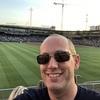
Ian Ostrom
Full Stack JavaScript Techdegree Student 10,332 PointsSolution for those in the FSJS Techdegree
Here's a possible solution for those who are in the FSJS Techdegree, using what we've learned in previous units.
const nameDiv = document.querySelector("#employeeList");
const nameUl = document.createElement("ul");
nameUl.className = "bulleted";
nameDiv.appendChild(nameUl);
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const employees = JSON.parse(xhr.responseText);
employees.forEach((e) => {
const li = document.createElement("li");
li.className = e.inoffice ? "in" : "out";
li.textContent = e.name;
nameUl.appendChild(li);
});
}
};
xhr.open("GET", "../data/employees.json");
xhr.send();
1 Answer

Mohamed El-Damarawy
11,294 PointsWell I used template literals instead of creating objects, check my solution below:
const officeStatusWidget = new XMLHttpRequest();
officeStatusWidget.onreadystatechange = () => {
if(officeStatusWidget.readyState === 4 && officeStatusWidget.status === 200){
let list = "";
const JSONresponse = JSON.parse(officeStatusWidget.responseText);
for( arrayItem in JSONresponse){
const obj = (JSONresponse[arrayItem]);
const status = obj.inoffice?'in':'out';
list += `<li class = '${status}'> ${obj.name} </li>`;
}
document.getElementById('employeeList').innerHTML += `<ul class = "bulleted">${list}</ul>`;
}
};
officeStatusWidget.open('GET','data/employees.json');
officeStatusWidget.send();
Yet I loved the forEach approach, goo job
Mia Filisch
16,117 PointsMia Filisch
16,117 PointsAh, much nicer!!! Thanks for sharing this. Whilst I appreciate the version used in the course was trying to presume as little previous knowledge as possible, I distinctly felt like "there's got to be a better way than this" whilst following along, and some things seemed downright questionable... (such as the redundant equality check in
if (employees[i].inoffice === true)
).