Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial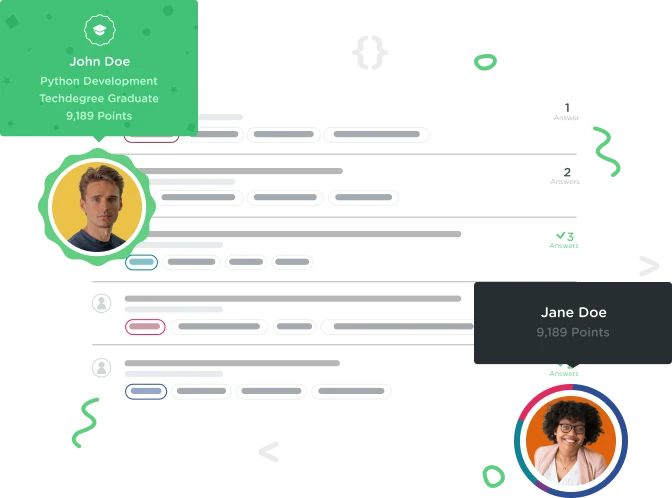
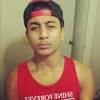
Dewan Payroda
1,954 PointsSKPhysicsContact Not Detecting categoryBitMask Collision
So I have my "Floor.swift" class below which is basically a bunch of walls. I have objects coming from the top of the screen and once the Floor and SKSpriteNodes collide, I'd like the SKSpriteNode to be removed. Below is my Floor class.
import Foundation import SpriteKit
class Floor: SKNode {
override init() {
super.init()
let leftWall = SKSpriteNode(color: UIColor.brown, size: CGSize(width: 5, height: 50))
leftWall.position = CGPoint(x: 0, y: 50)
leftWall.physicsBody = SKPhysicsBody(rectangleOf: leftWall.size)
leftWall.physicsBody!.isDynamic = false
self.addChild(leftWall)
let rightWall = SKSpriteNode(color: UIColor.brown, size: CGSize(width: 5, height: 50))
rightWall.position = CGPoint(x: 375, y: 50)
rightWall.physicsBody = SKPhysicsBody(rectangleOf: rightWall.size)
rightWall.physicsBody!.isDynamic = false
self.addChild(rightWall)
let bottomWall = SKSpriteNode(color: UIColor.brown, size: CGSize(width: 500, height: 10))
bottomWall.position = CGPoint(x: 150, y: -5)
bottomWall.physicsBody = SKPhysicsBody(rectangleOf: bottomWall.size)
bottomWall.physicsBody!.isDynamic = false
self.addChild(bottomWall)
// Set the bit mask properties
self.physicsBody?.categoryBitMask = floorCategory
self.physicsBody?.contactTestBitMask = objectCategory | pointCategory | lifeCategory
self.physicsBody?.collisionBitMask = dodgeCategory
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemted")
}
}
Then in my GameScene class under "func didBegin(_ contact: SKPhysicsContact)
" I wrote:
if (contact.bodyA.categoryBitMask == floorCategory) && (contact.bodyB.contactTestBitMask == objectCategory | pointCategory | lifeCategory) { contact.bodyB.node!.removeFromParent() print("COLLISION") }
But for some reason I'm not getting any detection what's so ever. I made my "Floor" class be a "contactTestBitMask" on each class of my objectCategory, pointCategory, lifeCategory. What am I doing wrong!? My other collisions are detected but not this one.I tried doing "let ourUnionOfBodies = SKPhysicsBody(bodies: [leftWall.physicsBody!, rightWall.physicsBody!, bottomWall.physicsBody!])" and following that I wrote on the next line "ourUnionOfBodies.isDynamic = false" but it still doesn't work? I don't see no collision detection they just fall right through.