Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial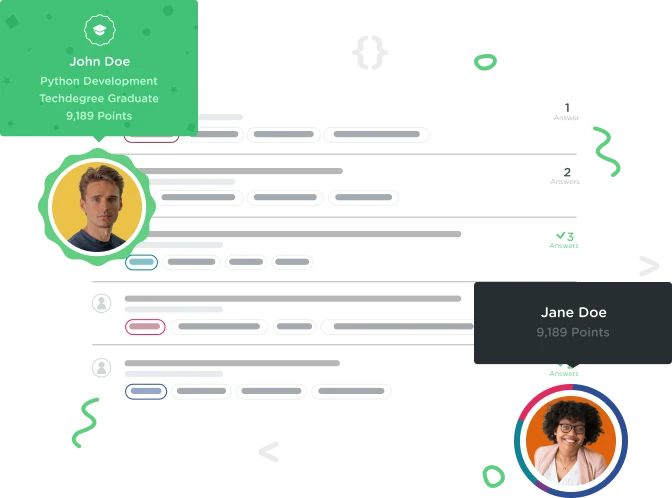

Alfredo Prince
6,175 PointsShould I even be using a condition inside the For loop?
It says that it does not see all of the facts listed. Any ideas?
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i=0; ++$i <=100;){
if (isset($facts) == $i){
echo $i;
}
}
4 Answers
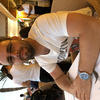
Matthew Underhill
20,363 PointsOK this code below works. I have just tried it on the challenge. Your for loop parameters are still technically the wrong way around, and you are missing a semi-colon on the second one.
The task says to echo the value after the number, but in your above code, you aren't echoing the value of the array element. You are only echoing the value $i
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for($i = 1; $i <= 100; $i++) {
echo $i;
if(isset($facts[$i])) {
echo $facts[$i] . "<br />\n";
}
}

Eric Drake
6,339 PointsAs your condition statement reads right now, you are echoing the value of $i when the the result of isset($facts) is equal to $i. The function, isset, only returns true or false (or 1 or 0) depending on whether or not a variable has been set. Because $facts is set and isset($facts) will return 1 and you are not checking if isset($facts) and $i are identical, your code will echo 1.
If I remember correctly, you are trying to echo the value associated with an index that has been set in the $facts array. Try something like the code below for your condition statement:
if (isset($facts[$i])) { //check if there is a value set for the given index echo $facts[$i]; //echo the value assigned to that index in the $facts array }

Alfredo Prince
6,175 PointsI tried that but to no avail.
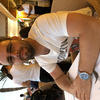
Matthew Underhill
20,363 PointsChange your for loop to this. I have also concatenated a new line to your echo command for better readability.
The square brackets with the counter variable means you are looking for if the array element with that key number is set.
for ($i = 0; $i <=100; $i++) {
if (isset($facts[$i])) {
echo $i . "\n";
}
}
There is nothing wrong with using conditionals inside for loops and foreach loops.
You had your parameters the wrong way around in the for loop by the way.

Alfredo Prince
6,175 PointsThis didn't work out. It said that Task 1 wouldn't pass after i tried
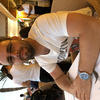
Matthew Underhill
20,363 PointsSorry I didn't realise this was related to a Treehouse task. I thought it was just something you were trying to output, as in that case it works fine.
Can you please copy the Treehouse question to this post so I can see the question. The criteria for the correct answer is obviously different.

Alfredo Prince
6,175 PointsSure thing, this is the task:
Use the function isset to test if the incremented value equals one of the keys in the $facts array. If there is a key that matches, display the value after the number.
Here is the code I am working with along with what I tried. The for loop passed the first task which was just to display numbers from 1 - 100.
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i=0; ++$i <=100;){
if (isset($facts) == $i){
echo $i;
}
}
Alfredo Prince
6,175 PointsAlfredo Prince
6,175 PointsOH, thank you for point that out. Now I see what i did wrong!