Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial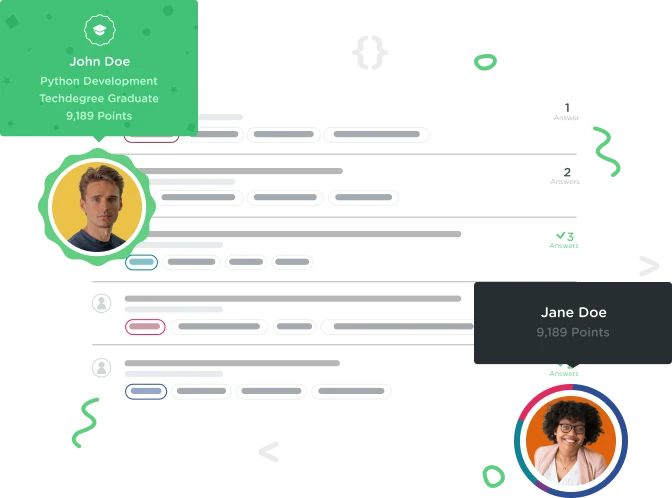

Natsai Mutyavaviri
6,656 PointsSelect the button with the ID sayPhrase and assign it to the button variable.
Not too sure what is required here
var button;
var input;
const sayPhrase = document.getElementByID('sayPhrase');
sayPhrase.addEventListener('click', () => {
button(input.value);
});
<!DOCTYPE html>
<html>
<head>
<title>Phrase Sayer</title>
</head>
<body>
<p><input type="text" id="phraseText"></p>
<p><button id="sayPhrase">Say Phrase</button></p>
<script src="js/app.js"></script>
</body>
</html>
9 Answers

Ajay Prasannan
16,002 PointsHi Natsai, you're very close, but all you need to do is make sure the result of document.getElementByID()
is assigned back into the button variable, as that's the one referred to in the function that appears later on. [Edit: I really should have said that "a click handler is assigned to the button variable later on."]
I ended up with the following, which passed the challenge:
var button = document.getElementById('sayPhrase');
var input;
button.addEventListener('click', () => {
alert(input.value);
});
Hope this helps!
PS. I expect a later task involves assigning the correct element to the input
variable.

Eddington Shayanowako
8,824 PointsHie Natsai and Ajay. To me the above code from Ajay is nor passing the test. I just copied as it is but its not passing

Ajay Prasannan
16,002 PointsHi Eddington, that's very odd. I just tried copying my snippet into the JS file and it definitely passes for me. Does the page give any error info?
Thanks,
Ajay

Alan Lane
9,156 PointsThank you jb30, that was a great catch, I did not even think that was any kind of issue! I figured ID would stay caps and not be a part of the camel case convention! It finally worked!!
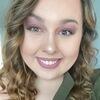
Brittani Kinzalow
Front End Web Development Techdegree Graduate 12,600 PointsFor task one of two answer is: var button = document.getElementById('btn-phrase');
For task two of two answer is: var input = document.getElementById('input-phrase');
You need to put the answer before the given text: button.addEventListener('click', () => { alert(input.value); });
You can't add both answers together on step one or you will get an error.

PAOLO Ruget
7,074 Pointsbless you

Alan Lane
9,156 Pointsvar button; var input; button = document.getElementByID('sayPhrase'); input = document.getElementByID('phraseText'); button.addEventListener('click', () => { alert(input.value); });
Why is this not working!! In the code above you have declared the variable twice, I got an error code for that, tried this and it tells me there is an undefined error...... Can someone point out where I went wrong!

jb30
44,806 PointsInstead of document.getElementByID
, try document.getElementById
with the last letter in lowercase.

Starky Paulino
Front End Web Development Techdegree Student 6,398 Pointslet button = document.getElementById('sayPhrase'); let input = document.getElementById('phraseText');
button.addEventListener('click', () => { alert(input.value); });
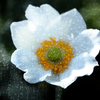
ellie adam
26,377 PointsHere is my solution
var button;
var input;
var button = document.getElementById('sayPhrase');
var input = document.getElementById('phraseText');
button.addEventListener('click', () => {
alert(input.value);
});
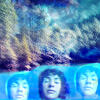
Amber Awada
3,080 PointsI've been trying to figure this out. I did everything above but im still getting a bummer:Cannot read property '1' of null

Alan Lane
9,156 PointsMe too! I copied the text exactly and it does not work!! Giving up!!

Eshwar N
4,568 Pointscheck this solution
var button = document.getElementById('btn-phrase');
var input;
button.addEventListener('click', () => { alert(input.value); });
Natsai Mutyavaviri
6,656 PointsNatsai Mutyavaviri
6,656 PointsIt worked! Thanks
Omar Ocampo
3,166 PointsOmar Ocampo
3,166 PointsThis code works perfect!!!
Thanks
Manoj Gurung
5,318 PointsManoj Gurung
5,318 Pointsthe question ask us to just do the 1st line right ?
Manoj Gurung
5,318 PointsManoj Gurung
5,318 Pointswhat question ask is different from the answer required- is this creating confusion