Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial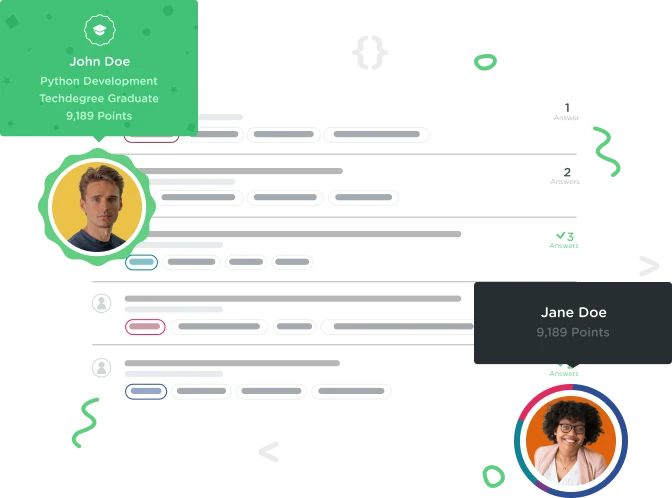

John Bergandino
8,455 PointsScrabblePlayer Code Challenge
I'm stuck on this challenge...I understand the concept of breaking the string down into an array of characters but I don't know how to have it loop through the characters and increment a counter if it matches a specific letter. Can someone please explain how to do this?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(String mHand) {
for (char letter: mHand.toCharArray()) {
if() {}
}
}
}
5 Answers

Stone Preston
42,016 Pointsyou are on the right track
the task states: Can you please add a method called getTileCount that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count
so we need to create a method that takes a tile as an argument, loop through the mHand member variable, and increment a counter if we come across the tile that was passed in to the method.
you are currently passing in the hand as a parameter, however you need to pass a tile which is a char. mHand is a member variable which is already available inside the class, there is no need to pass it in as a parameter. However, we will need to know which tile we want to get the count of, which is why the tile parameter is necessary.
you also need to create a counter variable before the loop. you can then use the for each loop to loop through the mHand converted to an array of character.
inside the loop, you can check if the letter in the hand is equal to the tile passed in to the method using the ==
oeprator. if it is, increment the counter.
// we pass in the tile we are looking to count in as the parameter
public int getTileCount(char tile) {
// counter used to count how many of the tiles we have in the hand. starts at 0
int counter = 0;
// loop through the hand
for (char letter: mHand.toCharArray()) {
// if the letter in the hand is equal to the tile parameter we need to increase the count by 1
if(letter == tile) {
counter += 1;
}
}
return counter
}

John Bergandino
8,455 PointsAwesome response!

Stone Preston
42,016 Pointsthanks! glad it helped

james white
78,399 PointsIt may have been a awesome response, but this getTileCount code (based off that response) is not passing for me (with the preview showing no syntax errors).
Any idea why?
public int getTileCount(char tile) {
int counter = 0;
for (char letter: mHand.toCharArray()) {
if(letter == tile) {
counter += 1;
}
}
return counter
}
//Here's the full code I'm using for ScrabblePlayer.java:
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
int counter = 0;
for (char letter: mHand.toCharArray()) {
if(letter == tile) {
counter += 1;
}
}
return counter
}
}
Java Objects Challenge Link: http://teamtreehouse.com/library/java-objects/creating-the-mvp/for-each-loop

John Bergandino
8,455 PointsYou need a semi-colon after "return counter"....sorry I should have pointed that out when I fixed it in my code.

james white
78,399 PointsThanks for the quick response (that got it finally passing)!
Could you take a look at the other question I just posted: https://teamtreehouse.com/forum/java-objects-challenge-fix-the-getlinefor-method

Daniel Abbott
16,780 PointsUgh... I was so close. That makes more sense that we can declare a new variable example: for([new variable type and name]: [then finish what your doing]) I know it's not my question but this helped a lot. I must have missed that part.