Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial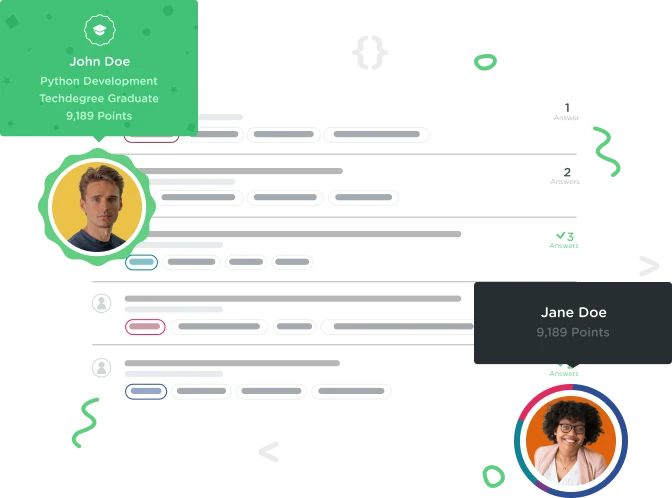
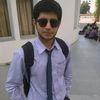
Neelesh Tewani
1,239 Pointsrequired no arguments ? i did not understand the task right?
.
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video;
course.add(video.getTitle("The Beginning Bits"));
// TODO(2): Add the newly created video to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
2 Answers
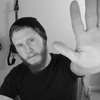
Jeremiah Shore
31,168 PointsYou haven't created a new object of type Video, you have only declared the variable "video" to be of type Video. In this case, the video object reference is null.
To create a new Video object:
Video video = new Video("Super Awesome Movie Title");
You are also trying to use the .add() method that your Course class does not have, when instead you should be adding the video to the member variable that course has that is a list of videos.
course.getVideos().add(1, video);
Additionally, the way you were using video.getTitle() is incorrect. When you call getTitle(), it has no arguments, but instead is used to return a String with the value of mTitle from your video object. You tried passing it a string, and it looks like you did that because you were trying to create a video. I answered how to do that first, by using the proper constructor of Video, which takes a String for a movie title.
Finally, even though the instructions are broken into two steps, you can technically condense them into one. To do so correctly:
course.getVideos().add(1, new Video("The Beginning Bits"));
I hope this helps. Let me know if you have any issues with the remaining tasks.
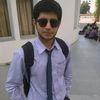
Neelesh Tewani
1,239 Pointsbut why you have put 1 in the argument //.add(1, new Video("The Beginning Bits"));
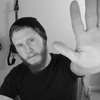
Jeremiah Shore
31,168 PointsIn the instructions it says to add the newly created video to the list as the second video. course.add(video) will add the item to the beginning of the list by default. You can use another add method available to lists that lets you specify the index of the video. Since this needs to be the second, and collections are 0-based, the index of the second position is 1.
add(int index, E element)
Inserts the specified element at the specified position in this list (optional operation).