Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial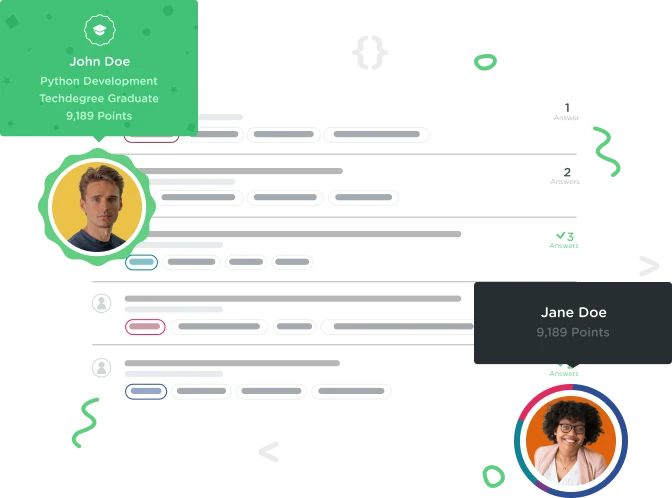

Zachary Junquera
1,792 PointsRegardless of what I do, the first task always fails when trying to get the second task to work. What am I missing?
By changing the value held in let inputValue to 'a' the first task automatically fails. Am I going about solving the first task wrong?
let input = document.querySelector('input'); let inputValue = input.value;
This was my solution to the first challenge, but if I change what's stored in inputValue in any way it fails the first challenge, negating whether or not it now passes the second challenge.
let input = document.querySelector('input');
let a = document.querySelector('a');
let inputValue = input.value + a.value;
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
2 Answers
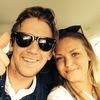
Jonathan Hermansen
25,935 PointsYou should store the value from textfield in task one, like this:
let inputValue = document.querySelector('input').value;
And then you could assign that value to the anchor tag in task 2, like this:
document.querySelector('a').innerHTML = inputValue;
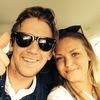
Jonathan Hermansen
25,935 PointsI'm not sure if I understand your mindset here. If you want to first create a variable (let input) of the input element, then create a new variable (let inputValue) with the value of the input variabel, you then need to add the value of inputValue to the a-element.
/*** Your first task ***/
let input = document.querySelector('input');
let inputValue = input.value;
/*** Second task can then be something like this ***/
document.querySelector('a').innerHTML = inputValue;
If you trying to achieve this outside treehouse, you have to add a click-event or something to get the value of the textbox, then assign it to the a-element. If you are trying to do this when textbox is empty (when opening a page), you get an empty value, since nothing is typed in yet.
By making a onkeyup-event, you can get the value of a textbox while typing in to it:
document.querySelector("input").onkeyup = function() {
document.querySelector("a").innerHTML = this.value;
}

Zachary Junquera
1,792 PointsI had just begun to realize this about doing it externally when I had to leave for work yesterday morning. That makes perfect sense, thank you!
As to the second task, I had been misunderstanding what was being asked. I thought they were asking me to change the value of what was in the variable inputValue to what was already in the element <a>, not have <a> take the value of inputValue.
Thank you for the help!
Zachary Junquera
1,792 PointsZachary Junquera
1,792 PointsI think I understand now that I was misunderstanding the intent. This solution actually stores the value of the input into <a>, rather than referencing an existing value in <a> and applying it to inputValue. Is this correct?
I'm also having trouble getting this to work outside of the Treehouse environment. When running the code in atom: let inputValue = document.querySelector('input').value; nets an empty string inside of inputValue. However, dropping the '.value' causes inputValue to have the correct value of '<input type="text" id="linkName>"'
Am I misunderstanding how this is working completely?