Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial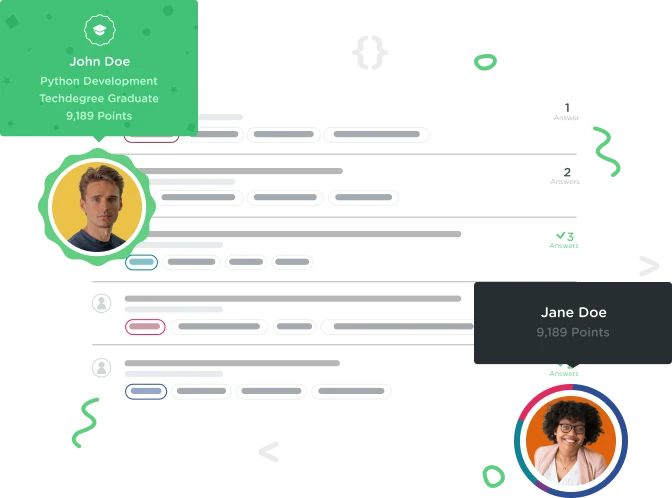
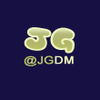
Jonathan Grieve
Treehouse Moderator 91,254 PointsRefresher on what Override is for
Hi JavaCoders.
This is what I've done for the Karaoke example so far. So far I'm realising I've probably learned more than I realised and (up to now) I've been able to follow the video well enough. I absolutely love how the course keeps building and reinforcing what we've learned even as the courses get more advanced Even packages are starting to make sense. :)
//import package
package com.teamtreehouse.model;
//new public class
public class Song {
//private unexposed variables - immutable variables. Can only get values not set them.
private String mArtist;
private String mTitle;
private String mVideoUrl;
//use constructor to initiliae variables from member variables.
public Song(String artist, String title, String videoUrl) {
mArtist = artist;
mTitle = title;
mVideoUrl = videoUrl;
}
//expose variables with "getter functions"
public String getTitle() {
return mTitle;
}
public String getArtist() {
return mArtist;
}
public String getVideoUrl() {
return mVideoUrl;
}
//Override Bases toString method -prepare instance to be used as a String.
@Override
public String toString() {
return String.format("Song: %s by %s", mTitle, mArtist);
}
}
package com.teamtreehouse.model;
import java.util.List;
import java.util.ArrayList;
public class SongBook {
//a new private interface List variable.
private List<Song> mSongs;
//constructor SongBook
public SongBook() {
mSongs = new ArrayList<Song>();
}
//public method, returns nothing. takes Song List as a parameter
public void addSong(Song song) {
mSongs.add(song);
}
//
public int getSongCount() {
return mSongs.size();
}
}
//import packages since main file is outside the package.
import com.teamtreehouse.model.Song;
import com.teamtreehouse.model.SongBook;
//new public class
public class Karaoke {
//static method named nain that takes array of args returns nothing.
public static void main(String[] args) {
//a new instance of a song from Song class
Song song = new Song("Michael Jackson",
"Beat It",
"https://www.youtube.com/watch?v=SaEC9i9Q0vk");
//a new instance of a songbook.
SongBook songBook = new SongBook();
//write to console
System.out.printf("Adding %s %n", song);
songBook.addSong(song);
System.out.printf("There are %d song(s). %n", songBook.getSongCount() );
}
}
But before I tear myself away from the computer screen and take a break I wanted to ask about Override is this is the only topic in that video I'm still struggling with. Why do we use the @Override annotation and what does it do? Thanks :)
1 Answer
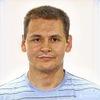
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 Points@Override annotation overrides method in Child
that was inherited from Parent
.
Annotation itself is important to tell the compiler that you are actually overriding method inherited from Parent
.
Take a look here for more: http://stackoverflow.com/questions/94361/when-do-you-use-javas-override-annotation-and-why
One of the good quotes from there is:
"...you should use the @Override annotation if the lack of a method with the same signature in a superclass is indicative of a bug."