Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial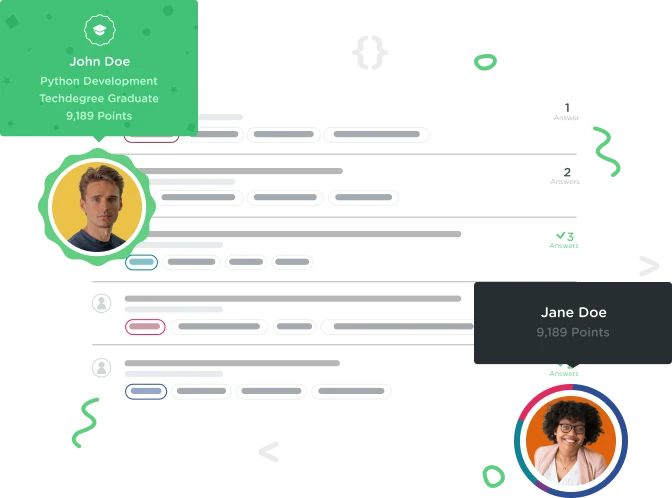
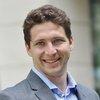
Jacques Kleynhans
Courses Plus Student 4,225 PointsReally battling to grasp this particular concept of concatenation. converting and then combining.
Trying to figure out where I am going wrong. I have tried a few different methods, but clearly I am missing some fundamentals.
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase();
userName + "#" + lastName;
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
2 Answers

Jacob Mishkin
23,118 PointsIts totally cool, let break this down! take a look at the code below, and we will go over it.
//your code
var id = "23188xtr";
var lastName = "Smith";
//issue spot
var userName = id.toUpperCase(); //remove the semicolon everything needs to be joined.
userName + "#" + lastName; //last name also needs to be uppercase as well use toUpperCase() here as well as id
// no need to concatenate userName
//new code
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase() + "#" + lastName.toUpperCase();
you are very close in this, the key here is to use the "+" to concatenate the two variables plus the string "#". remember if you use a semicolon you are ending the line, its like a "break" or "stop in Morse code.
second, you are putting the variables id and lastName inside the variable userName. UserName does not need to be called because you are putting the other variables inside it. and use the "+" operator and remember to use the toUpperCase() method and you should be good to go.
if you have any more questions pleases feel free to help. That's what we are here to do.

Jacob Mishkin
23,118 PointsRich,
Awesome!! well Jacques, there you go!
Jacques Kleynhans
Courses Plus Student 4,225 PointsJacques Kleynhans
Courses Plus Student 4,225 PointsAwesome, Thanks! Knew it was going to be something obvious! I do have a question though, is there no way to make the whole 'var userName' all 'toUpperCase' instead of having to do it individually?
Jacob Mishkin
23,118 PointsJacob Mishkin
23,118 PointsI don't think so, because in this scenario you are concatenating a string, as well as variables, But I'll look into it and find out.
Jacques Kleynhans
Courses Plus Student 4,225 PointsJacques Kleynhans
Courses Plus Student 4,225 PointsThanks again Jacob!
Jacob Mishkin
23,118 PointsJacob Mishkin
23,118 PointsNot a problem! any time Jacques.
Rich Donnellan
Treehouse Moderator 27,721 PointsRich Donnellan
Treehouse Moderator 27,721 PointsIt is possible — I had success converting the output using one
toUpperCase()
method:Jacques Kleynhans
Courses Plus Student 4,225 PointsJacques Kleynhans
Courses Plus Student 4,225 PointsYeah! Thanks Rich!!! Gotta love those ( ) ...