Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial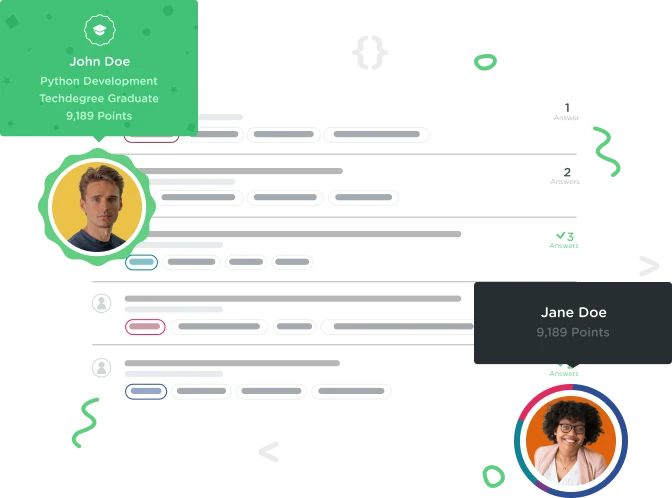

Jutyar Nerway
1,242 PointsReading location from multiple users with unique ID using Swift 4 and Firebase
Hello!
so I've been struggling with this one for a two days now. I've searched everywhere and haven't really found an answer that could help me. Maybe I'm using the wrong keywords.
Here is the issue.
I have this data in my Firebase Realtime Database
-users VZUFNaLJ6YN5aqIDaFGmxWKGYNc2 -location -latitude: 123 -longitude: 123 RXUFNaLJ6OI7G57DaFGmxWKHG76T -location -latitude: 321 -longitude: 321 And the output I'm hoping to get is this
latitude: 123
longitude: 123
latitude: 321
longitude: 321
I really don't have a working code to post here, I was hoping if someone could help me understand what needs to be done. Thank you.
What I have so far is this
// Read location from all user func globalCoor(){ ref.child("location").observeSingleEvent(of: .value) { (snapshot) in let locationData = snapshot.value as? [String: Any] print(locationData) } }
1 Answer
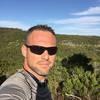
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsSo you are just trying to get the data out of Firebase correct?
You need to model your data with a couple of structs first. Judging by your data it will be as [String: [String: Any]].
Keep in mind I haven't ran this code but this should get you going:
struct User {
userID: String
longitude: Int
latitude: Int
// Initialize below. You will most likely have to convert string to Int
init?(userKey: String, dict: [String: Any]) {
let userID = userKey
guard let longitude = dict["longitude"] as? Int,
let latitude = dict["latitude"] as? Int
}
self.userID = userID
self.longitude = longitude
self.latitude = latitude
}
}
Next you will need a user snapshot struct which will have a object 'users' which is an array of 'User'.
struct userSnapshot {
let users: [User]
init?(with snapshot: DataSnapshot) {
var users = [User]()
guard let snapDict = snapshot.value as? [String: [String: Any]] else { return nil }
for snap in snapDict {
guard let user = User(userKey: snap.key, dict: snap.value) else { continue }
users.append(user)
}
self.users = users
}
So now the data will be modeled into one object which is an array of 'User' and the next object will be the individual user so everything is separate. I think this is what you are trying to accomplish.
Now when you get your data from Firebase it will be something like this.
// Instead of this
locationData = snapshot.value as? [String: Any] print(locationData) } }
// Do this
guard let userSnapshot = userSnapshot(with: snapshot) else { return }
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsBrandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsAlso it is better to keep data about the user in the user node of your database such as name etc and then have a separate node which is location. Research relational database. You want to keep it shallow and not nested as much as possible.
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsBrandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsAlso make sure to check the markdown sheet so your code is readable when you post. It should look like this:
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsBrandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsDid this help you out?