Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial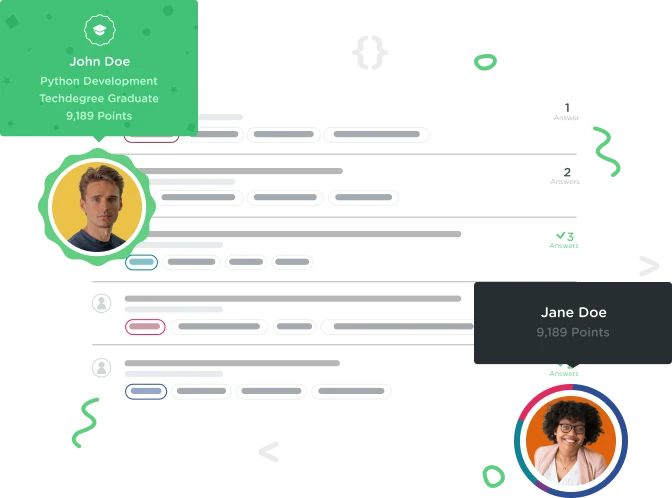

Mark Kohner
1,121 Pointsrandom.choice with iterables.. comprehension issues
I am frustrated by these, I am getting stuck but I don't have any way I can find to understand the material. I am going to type through my mental struggle... perhaps it will help me understand it, or help others with similar issues in comprehension.
I think I get the syntax fairly well, but I do not comprehend fully, even though I have run through some examples, using these iterables in functions.
Here is the question:
create function random_item, that takes a single argument, generate a random number between 0 and length of the argument -1. Return the random index.
EXAMPLE
random_item("Treehouse")
The randomly selected number is 4.
The return value would be "h"
import random def random_item(fruits): random.randint(0, len((fruits)-1) return fruits[random_item]
for the return value, I am lost.
I understand that: import random myNumber = random.randint(1,20) print(myNumber) is going to return that random number. But I do not fully understand how this ties in with these iterables.
Even using them is confusing to me.
for example,
def random_items(#mental):
mental this variable starts my confusion. Its a local scope, a variable within a function, its destroyed outside of the function. I think that is correct and that I understand it. If we referenced mental outside of the function it wouldn't work. Is t his correct? Is this the same as a local variable in a function, or is it being the 'iterator' causing it special properties?
ie:
def easyFunction(): spam = 42 print(spam)
returns 42 when function is called
print(spam) outside of function = undefined / error
is this iterable variable the same ?
How do I type in code blocks? I do not see formatting help and tabs don't work. Anyways:
def random_items(fruit):
random.randint(0, len((fruits) - 1)) #I believe that is correct, its a bit awkward though. ###otherwise, I suppose we could assign a variable to equal this value . Perhaps this is the solution to my problem. Sounds like it. Is there a way to reference this value without a seperate local variable? # ##
indexValue = random.randint(0,len((fruits) - 1 ))
return fruits[indexValue]
this still did not work. I am not really understanding this, as what exactly is our 'fruit' #variable? Its a string or a list? so we are attempting to return the item from our non- # existant list /string at the index value. What is an iterable member? How can we return
a value for a non-existant string/list ?
# EXAMPLE
# random_item("Treehouse")
# The randomly selected number is 4.
# The return value would be "h"
import random
def random_item(fruits):
indexValue = random.randint(0, len((fruits)-1)
return fruits[indexValue]
unfortunately I am still stuck but I will try to work on it... if anyone takes the time to go through that mess, thanks in advance!
3 Answers
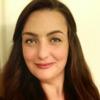
Jennifer Nordell
Treehouse TeacherI took time to read through your mess as you call it. And I'm of the opinion that you understand it a lot better than you give yourself credit for. You're frustrated and I totally get that! Anyone who has ever programmed does
Would it make you feel better to know that your giant mistake here is one extra parentheses? Seriously. That's all. There's no giant logic flaw here.
But for a little clarifcation: you ask what an iterable member is. It is simply an item inside an iterable. Now that can be a letter inside a string or an item in an array etc.
As for the formatting: all I can tell you here is to be careful with it. I generally just use the space bar. Annoying, I know.
You ask how we can return a value for a non-existant string/list. We can't. We'll get compiler errors. But in the definition of the function ... see that fruits there? It is the piece of information coming into the function. It's being sent there by another piece of code. In this case, we have no idea what that information will be. Why is that? Because Treehouse is running your code and sending in the iterable. Then whatever that iterable was will be run through the code. And we'll use the name fruits, because that's what you've chosen. And yes, when the function exits, fruits will be gone again because it's local to that function. I've yet to come across anything you haven't understood yet
Here's your modified code that passes:
import random
def random_item(fruits):
indexValue = random.randint(0, len(fruits)-1)
return fruits[indexValue]
Hang in there! You're doing much better than you think you are
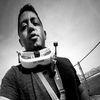
hector villasano
12,937 Pointsyour in the right path, but your fruits variable is not a list but a string .
So you must first convert the string to a list before finding the length!
Example
fruit = "hello"
fruits_length = len(list(fruit))
output: 4
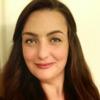
Jennifer Nordell
Treehouse TeacherI don't agree. The code I listed works and the challenge says this: "from an iterable (like a list or a string)."
We have no way to know whether Treehouse is sending in a list or a string or an array.
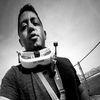
hector villasano
12,937 Points#EXAMPLE
#random_item("Treehouse")
#The randomly selected number is 4.
# The return value would be "h"
This is part of the challenge
random_item is a string
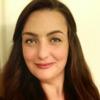
Jennifer Nordell
Treehouse TeacherYes, in the example in the comment it is a string. But look again at the challenge instructions. It explicitly states an iterable. Not necessarily a string.
hector villasano
12,937 Pointshector villasano
12,937 Pointshow do you add a code block like that?
maybe he forgot to add the list() function?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHector, I've already posted a working solution. The list() is not needed nor a conversion to a string. Take a minute and read/try my solution. The problem is he has an extra open parentheses. That's all.
As for posting code blocks: There's a markdown cheatsheet link at the bottom of this page that will show you how to do it.
Abdallah El Kabbany
2,042 PointsAbdallah El Kabbany
2,042 Pointsever since i started learning how to program and i picked python out of all, i havent been able to go through a challenge on my own i have to go through community. does that mean i am not learning it right or maybe i dont fully understand the concept? the thing is that i feel that i understand but sometime things are written in a way i find really hard to understand. i dont know what to do.
for example why do you have return fruits and then brackets around the variable why not just the variable. things like that confuses me.
even when i took that could it didnt work. lol hahahaha shall i just quit?
check it out not working:
EXAMPLE
random_item("Treehouse")
The randomly selected number is 4.
The return value would be "h"
import random
def random_item(fruits): indexvalue= random.randint(0, len(fruits)-1) return fruits.indexvalue
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherAbdallah El Kabbany Well absolutely don't quit! As far as I know, that's never been the answer to anything
Well, let's take a closer look at this. Let's imagine that this code isn't taking a string. Let's say it's taking a whole list of strings that contain a bunch of fruits that we need for our fruit salad.
Let's imagine that we are are going to call this random item list and have it pick a random fruit from our list (for some odd reason). So the fruits we're sending in are: Apple, Banana, Pineapple, Kiwi, Mango, Papaya, Grape, Orange, and Pear. We want it to pick one of those. Now we're going to send these in in an array which will look like this ["Apple", "Banana", "Pineapple", "Kiwi", "Mango", "Papaya", "Grape", "Orange", "Pear"]. So we have 9 fruits. That's the length of our fruits list. But humans... we start counting from 1. Computers generally start counting from 0.
So let's take 9 boxes and start setting them up. But we're going to label them starting with 0. The boxes are labeled like this and contain the following fruit:
Box 0 = Apple Box 1 = Banana Box 2 = Pineapple Box 3 = Kiwi
Box 4 = Mango Box 5 = Papaya Box 6 = Grape Box 7 = Orange Box 8 = Pear
So we still have our 9 fruits, but because they start at a box named 0 the last box is box 8.
Now we're going to get a random number. And our random number is 3! So we are going to get out the fruit in box 3 and return it to the ... chef, I suppose
And we do this by writing:
return fruits[3]
This will return a kiwi (or the item in box 3 to whoever requested it. This is just how we write it in python (and actually a few other languages as well).
I hope this helps somewhat! Let me know if it was clear as mud
P.S. The reason your code is failing is because you're missing the square brackets. The dot notation you're trying to use is something we use on objects later on down the line, and we're not really there yet