Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial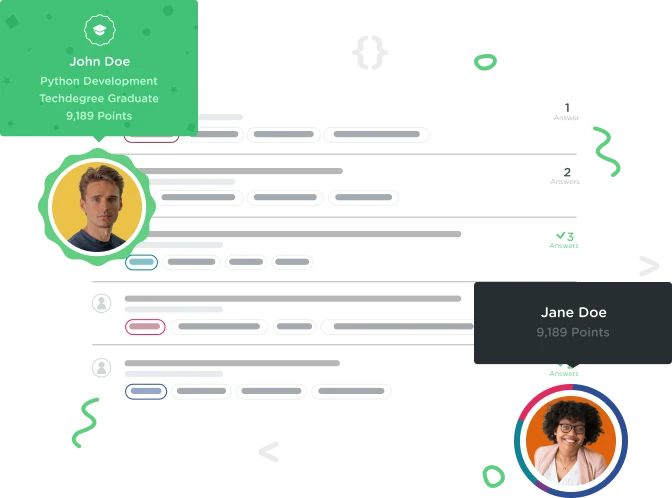

Alejandro Hernandez
PHP Development Techdegree Student 1,209 PointsQuestion about sorting an array in reverse.
$colors = array("Red", "Orange", "Yellow", "Green", "Blue", "Purple", "Black"); Which function will sort the array so the first element is "Yellow"?
The correct answer should be rsort, but this answer is not in the multiple choice answers. Could someone please clarify why I would be wrong or correct? The only answers I see is:
asort - sort associative arrays in ascending order, according to the value ksort - sort associative arrays in ascending order, according to the key krsort - sort associative arrays in descending order, according to the key arsort - sort associative arrays in descending order, according to the value sort - sort arrays in ascending order
The first four choices are key value pairs, the array is not a key value pairs. rsort is missing, it should be that.
1 Answer
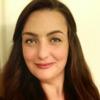
Jennifer Nordell
Treehouse TeacherHi there, Alejandro Hernandez! Even though this is not an associative array, it does have "keys". You may also know them as indexes. It's looking for the answer arsort
. This would sort the array in reverse, but the big difference here is that it maintains the key/index association. Even though "Yellow" would appear at the beginning of the array, it would still maintain the index of 2.
Here's some example code:
<?php
$fruit_basket = ['apples', 'bananas', 'kiwi', 'pineapple'];
arsort($fruit_basket);
echo "\nMaintaining keys:\n";
foreach($fruit_basket as $key=>$value) {
echo "$value is at an index of $key\n";
}
rsort($fruit_basket);
echo "\nNot maintaining keys:\n";
foreach($fruit_basket as $key=>$value) {
echo "$value is at an index of $key\n";
}
The output is:
Maintaining keys:
pineapple is at an index of 3
kiwi is at an index of 2
bananas is at an index of 1
apples is at an index of 0
Not maintaining keys:
pineapple is at an index of 0
kiwi is at an index of 1
bananas is at an index of 2
apples is at an index of 3
Notice that they are printed out in the same order, but the "keys" or indexes have changed in the second one. Either rsort
or arsort
is a correct answer for this question, but since rsort
is not available, the only correct answer left is arsort
. I think the biggest confusion is between normal arrays and associative arrays. A normal indexed array is pretty much exactly like an associative array, except that the keys aren't anything we define ourselves. The PHP interpreter takes our list of items, creates a range of integers such that the "keys"/indexes start at 0 and go up to the length - 1 of our list of items. In associative arrays, we name the keys ourselves.
Hope this helps!