Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial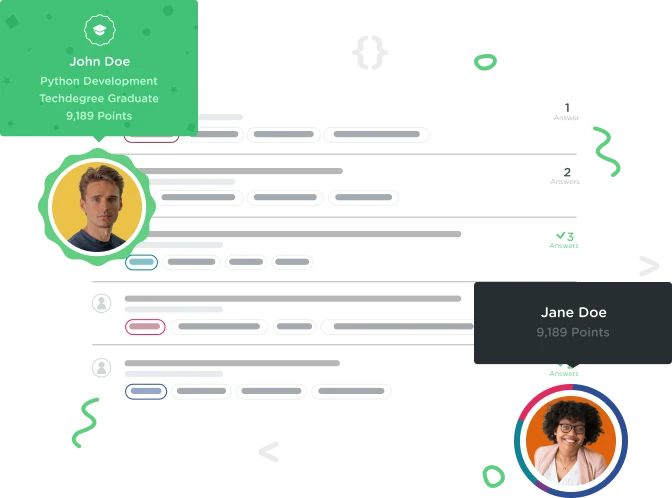
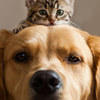
Devin Scheu
66,191 PointsPython Help
Question: Create a variable named players that is an re.search() or re.match() to capture three groups: last_name, first_name, and score. It should include re.MULTILINE.
Code:
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r'''
(?P<name>(?P<last>[-\w ]*,\s(?P<first>[-\w }+))\t # last and first names
(?P<score>\(?\d{2}\)?) # score
''', re.MULTILINE)
print(players)
1 Answer
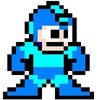
Robert Richey
Courses Plus Student 16,352 PointsHi Devin,
That's a really good attempt. Let's try and build it incrementally and see if that helps.
Create a variable named players that is an re.search() or re.match() to capture three groups: last_name, first_name, and score. It should include re.MULTILINE.
Regular expression functions have this basic syntax:
re.search(pattern, string[, options])
With this, we can build our skeleton function:
players = re.search(r'', string, re.M)
We have to capture 3 specific groups: last_name, first_name, and score, so let's set those up next.
players = re.search(r'(?P<last_name>)(?P<first_name>)(?P<score>)', string, re.M)
Finally, we need to figure out how to pattern match each of those groups. One thing to keep in mind is that the challenge is giving us a player with - what amounts to be - two last names and two first names. As ridiculous as that is to say, that's a practical way of thinking about it when writing the pattern.
For last names, one or more word characters, followed by an optional space, followed by an optional sequence of one or more word characters. In code, that looks something like this:
players = re.search(r'(?P<last_name>[\w]+\s?[\w]+?) # ...
I'll leave the rest for you to finish. I hope this was a helpful nudge in the right direction!
Cheers
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsOkay so this is what I got, i'm getting an error: unexpected end of regular expression
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsEDIT: I didn't read your statement all the way through, now i'm getting error: players doesn't seem to be an rgex object
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsPython doesn't know when one group ends and another begins.
Between these groups, we need to include any characters that will match but will not be selected. After the last name, we can expect to see a comma followed by a space.
players = re.search(r'(?P<last_name>[\w]+\s?[\w]+?),\s(?P<first_name> # ...
After the first name, we can expect to find a colon followed by a space. And finally, for the score, I'd try to match one or more digits. There are no parentheses in the string provided, so I wouldn't try to optionally match those.
To help make the pattern easier to read, we can break it up onto multiple lines if we pass the verbose flag
re.VERBOSE
orre.X
.Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsThanks Robert! I understand now.