Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial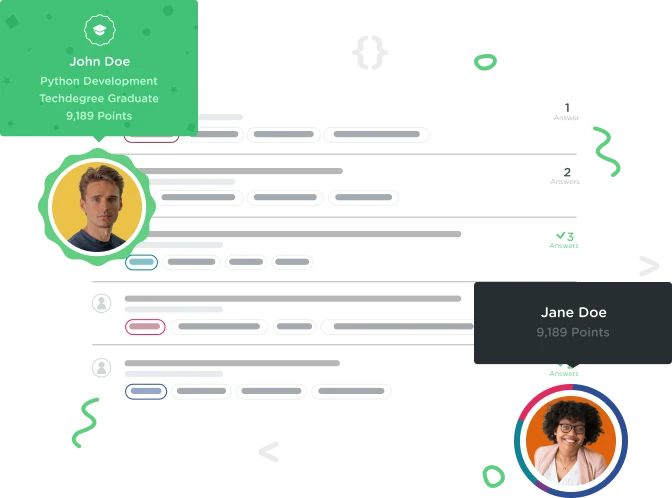

Teri Dent
11,515 PointsProperties clarification
Is there any real difference between the term properties and the various kinds of properties (stored, type, computed, etc)? I think that they are being used interchangeably but I am not quite sure. I several tutorials I have seen on the web they talk about using a property in the viewController to access storyboard items (e.g. IBOutlets) and I am trying to clarify the meaning of the term compared to what Passan has mentioned in his OOP swift tutorials here.
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsFrom the Apple Docs - Properties:
Properties associate values with a particular class, structure, or enumeration. Stored properties store constant and variable values as part of an instance, whereas computed properties calculate (rather than store) a value. Computed properties are provided by classes, structures, and enumerations. Stored properties are provided only by classes and structures.
Ok, there is a lot of information in this paragraph, so here is a tiny example struct.
struct PropertiesOverview {
// This is a *stored property*, in this case it is *mutable*
// which means it can be changed later. Also, it's initial
// value is 1. Type declaration Int is not mandatory, as
// Swift can infer the *type* (note that Int is a type).
// var anInteger = 1 would work as well
var anInteger: Int = 1
// This stored property is a constant, it's value cannot be
// changed at a later point. As pointed out before, Swift
// can infer the *type* String, so we do not explicitly need
// it here
let aString = "Hello"
// This is a *computed* property, that is no value is actually
// stored, but "calculated" or constructed. In this special case,
// it only has a *getter*. The *type* is String.
var anotherString: String {
return "Hello"
}
// This is also a *computed* property, this time it has a getter
// and a setter. It also does not store values directly, but rather
// gets and sets the value of the above declared **anInteger**.
// Note that "newValue" is, as the name suggests, the variable
// name of the new value that Swift provides by default within
// the setter.
var anotherInteger: Int {
get { return anInteger }
set { anInteger = newValue }
}
// This is a *type property*, that is you can access this **without**
// having an instance of `PropertiesOverview`. You can access it via
// dot notation: `PropertiesOverview.pi`
static let pi: Double = 3.14159265359
// ...
}
I really recommend reading the Apple Docs mentioned above, they provide valuable information and feature excellent examples.
Hope that helps :)
Teri Dent
11,515 PointsTeri Dent
11,515 PointsThanks for your clarification. I was on that doc from Apple trying to answer my own question, but even it misses the mark. What I have is the term "property" or its plural being used to describe something you put in to a view controller to allow reference to the object (I think). The second term "property" is of the group you described above; never found by itself (e.g. Stored property). I guess I am confused as it seems that the term "property" means something by itself (or possible it does not) compared to stored properties, computed properties, etc.
In short: without using a qualifier of any kind, does the term "property" have a mean unto itself that differentiates it from stored properties, computed properties, etc?
Thanks for all of your time
Martin Wildfeuer
Courses Plus Student 11,071 PointsMartin Wildfeuer
Courses Plus Student 11,071 PointsProperty is the generic term. We talk about about a property if it is a value / variable within a class, struct or enum. In detail there are stored properties, computed properties etc., but after all, they are all properties.
It does not matter what type the value stores, being it an object or a primitive like integer: it is a property. There is no two groups as such. The top level is property, divided into the different kinds of properties.
An IBOutlet, for example, is just a property as anything else. The IBOutlet annotation only tells Xcode that you want to see the property in Storyboards and XIBs so you can connect it. But it is a variable in a class. No differentiation.
Does that make sense?