Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial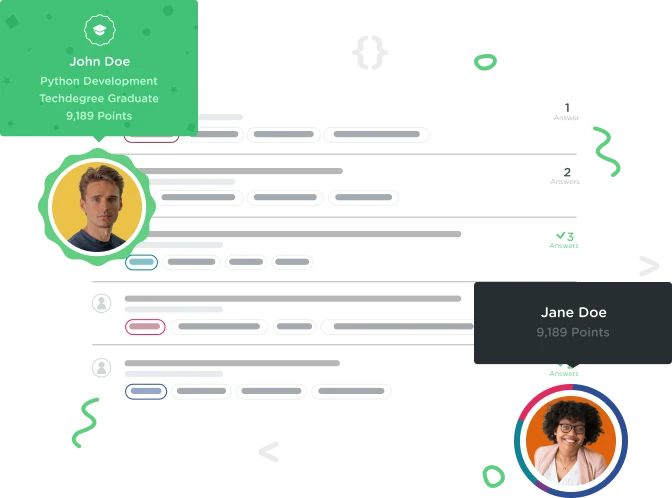
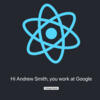
drew s
Python Development Techdegree Graduate 19,491 PointsProgram is not running
I need help running this program
public class CircularLinkedList {
//Both of this variable are pointing to null as default unless you asign anything to them
//define the first variable
private Node first;
//def last variable
private Node last;
//create constructor that gives this variable values
public CircularLinkedList(){
first = null;
last = null;
}
//this method retunrn true if its empty
private boolean isEmpty(){
return first == null;
}
//implement the insert first method
public void insertFirst(int data){
//iniitilize a new Node
Node newNode = new Node();
//give this node some data
newNode.data = data;//that's gonna be the data that pass in parameter
if(isEmpty()){
last = newNode;//we assign the end of the list to be the the newNode we just created.
}
//this newNode next field should reference whatever the old first was referecing by assigning first to newNode.next
newNode.next = first;//newNode is being assign to reference old first
first = newNode;//first place, first node that's connected to the engine of train
}
//this methid insert at the last of node
public void insertLast(int data){
//create new node
Node newNode = new Node();
//assign data that pass in to the parameter
newNode.data = data;
if(isEmpty()){
first = newNode; //if true then first newNode will be the first
}else { //if not empty
last.next = newNode;//next value of the last node will point to the new node.
last = newNode; //make the new node we created be the last one in the list
}
}
//delete first method
public int deleteFirst(){ //delete something at the beginning of the list
int temp = first.data;//whatever the data in the first node
//this means when we only have a single node
if(first.next == null){
//since we're deleting that one and only node
last = null;//then of course last should point to null
}
//first node always point to the next node
first = first.next;//first will point to old's next value
return temp;
}
//display lists method
public void displayList(){
System.out.println("List (first --> last)");
Node current = first;
while(current != null){
current.displayNode();
current = current.next;
}
System.out.println();
}
}
public class App {
public static void main(String[] args){
CircularLinkedList myList = new CircularLinkedList();
myList.insertFirst(1);
myList.insertFirst(2);
myList.insertFirst(3);
myList.insertFirst(4);
myList.insertLast(5);
myList.displayList();
}
}
Chris Freeman
Treehouse Moderator 68,426 PointsChris Freeman
Treehouse Moderator 68,426 PointsReposted into Java section for better response.