Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial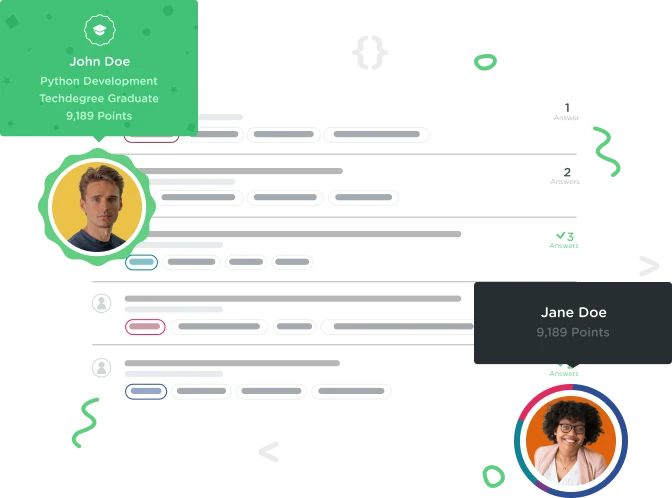
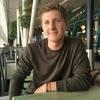
Sondre Fjellving
4,700 PointsProbably a really simple answer to this, but my head is stuck
I need to edit the conditional statement, so that when the input value from the text field matches with any of the indexes in the law variable (law variable is contains a list of li's), it will make the text go bold in the sentence with the same index.
I think I explained this poorly. So if you have a hard time understanding what I'm trying to ask for, check the task's question. There should be a direct link on this page to the task. Thanks in advance :)
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if () {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer

andren
28,558 PointsThere is indeed a pretty simple answer to this task, though it's not rare for students to get stuck as it can be a bit hard to figure out at first.
Within this loop there are two indexes at play, the i
variable which ensures the loop only runs once for each li
element and ensures a different li
is pulled out every loop. And the index
variable which stores the index that the user wants to change.
To make this code work you actually just need to check if the two indexes are equal, like this:
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (i === index) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
If i
and index
match then you know that the currently pulled out li
has an index matching the one the user input. Since i
is actually what is used as the index to pull li
elements out of the laws
list.
Sondre Fjellving
4,700 PointsSondre Fjellving
4,700 PointsHey man! Sorry for the late answer. I tried your solution now, and no surprise; it worked like a charm! Thank you so much for taking your time to give me such an in depth explanation, muchos appreciatos mi amigo!! I actually understand it now because of you.