Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial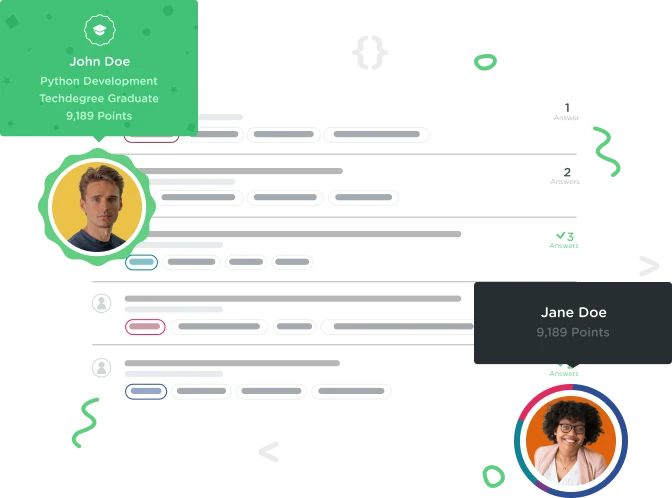
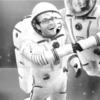
Wouter Willebrands
iOS Development with Swift Techdegree Graduate 13,121 Points(Possible) Parsing error when trying to decode data from API call
I have a generic method that makes a request for a page of data from TheMovieDB. The top layer of the JSON looks like:
{ "page": 1, "total_results": 10000, "total_pages": 500, "results": [ some array of dicts ] }
I have a Page object:
struct Page<T: Codable>: Codable { let page: Int? let totalResults: Int? let totalPages: Int? let results: [T]? }
I'm using a generic method so it can be used for other paged data object as well that looks like:
static func getPage<T>(url: URL, completionHandler completion: @escaping (Page<T>?, Error?) -> Void) {
let request = URLRequest(url: url)
let task = PageHandler.session.dataTask(with: request) { data, response, error in
DispatchQueue.main.async {
guard let httpResponse = response as? HTTPURLResponse else {
completion(nil, MovieDBError.requestFailed)
return
}
if 200...299 ~= httpResponse.statusCode {
print("Status Code: \(httpResponse.statusCode)")
if let data = data {
print(data)
do {
let results = try self.decoder.decode(Page<T>.self, from: data)
print(results)
completion(results, nil)
} catch let error {
print("Error Exit 4")
completion(nil, error)
}
} else if let error = error {
print("Error Exit 5")
completion(nil, error)
}
} else {
print("Status Code: \(httpResponse.statusCode)")
completion(nil, MovieDBError.invalidData)
}
}
}
task.resume()
}
The print logs including the error I'm getting is as follows:
Status Code: 200 45885 bytes Error Exit 4 error: dataCorrupted(Swift.DecodingError.Context(codingPath: [CodingKeys(stringValue: "results", intValue: nil), _JSONKey(stringValue: "Index 0", intValue: 0), CodingKeys(stringValue: "popularity", intValue: nil)], debugDescription: "Parsed JSON number <29.108> does not fit in Int.", underlyingError: nil))
At first I thought it might be that there is a mismatch in type (string/int) as the debugDescription suggest. I tried solving this by removing the "." from the totalPages value. Although as the print statement "Error Exit 4" suggests the error occurs earlier.
Anyone able to help me solve this issue? Also, please let me know what and if more information is needed to be able to solve this problem.
Thanks!