Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial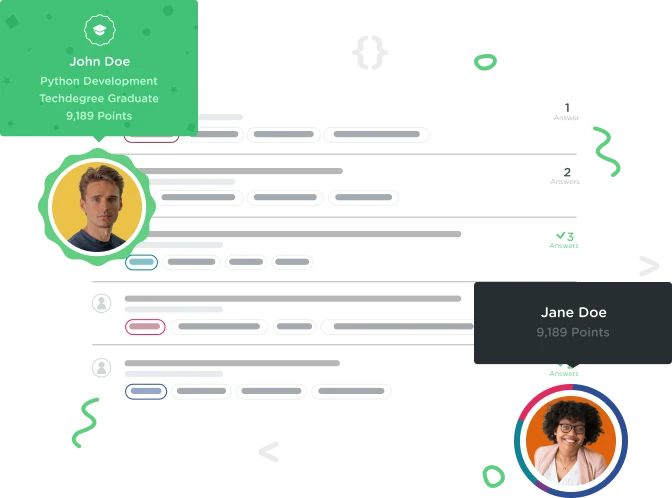

ashmagupta
6,595 PointsPls help me. I am stuck here like forever.
This is the error showing on preview . ./com/example/BlogPost.java:31: error: cannot find symbol return mCreationdate.compareTo(blogpost.mCreationdate); ^ symbol: variable mCreationdate location: variable blogpost of type BlogPost ./com/example/BlogPost.java:31: error: cannot find symbol return mCreationdate.compareTo(blogpost.mCreationdate); ^ symbol: variable mCreationdate location: class BlogPost 2 errors
package com.example;
import java.util.Date;
// Assuming public class implements comparable//
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
// producing compareTo method//
public int compareTo(Object obj){
BlogPost blogpost = (BlogPost) obj;
if (equals(obj)){
return 0; }else
{ return 1;}
//cast the object to file//
//Chronologically order creationdate//
int dateCmp = mCreationDate.compareTo(blogpost.mCreationDate);
if (dateCmp == 0) {
return mCreationdate.compareTo(blogpost.mCreationdate);
}
return dateCmp;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
2 Answers

andren
28,558 PointsThere are a couple of issues:
The first is in your first if statement:
if (equals(obj)) {
return 0; }
else {
return 1;
}
You tell Java to either return
0 or 1 based on the comparison, that means that the method will always return a value at this point, when a method returns a value it stops running. Which means that all of the code below this if
statement will never be run in any situation.
This can be fixed by simply removing the else
statement.
The second issue is that the mCreationDate
field is private, that means that you can't directly access it from the cast BlogPost
object. You have to use the getCreationDate
getter that has been setup to retrieve that field.
The third issue is that you misspell mCreationDate in this line:
return mCreationdate.compareTo(blogpost.mCreationdate);
You forgot to capitalize the D in the first mCreationDate
.
If you fix all of those issues like this:
package com.example;
import java.util.Date;
// Assuming public class implements comparable//
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
// producing compareTo method//
public int compareTo(Object obj){
BlogPost blogpost = (BlogPost) obj;
// Remove else statement
if (equals(obj)){
return 0;
}
//cast the object to file//
//Chronologically order creationdate//
// Change mCreationDate to getCreationDate()
int dateCmp = mCreationDate.compareTo(blogpost.getCreationDate());
if (dateCmp == 0) {
// Change mCreationDate to getCreationDate() and fix typo
return mCreationDate.compareTo(blogpost.getCreationDate());
}
return dateCmp;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
Then your code will work

ashmagupta
6,595 PointsThanks, Yep, its passed.
ashmagupta
6,595 Pointsashmagupta
6,595 PointsHi Andren, Thanks for replying. When i remove else from the if statement, the code didn't pass and it showed more error.
andren
28,558 Pointsandren
28,558 PointsThat's odd, I tested the code that I posted above before submitting the answer so it should work. The challenges can sometimes be a bit buggy, so maybe try to restart the challenge and then copy and paste in the code from my answer. That should work correctly.