Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial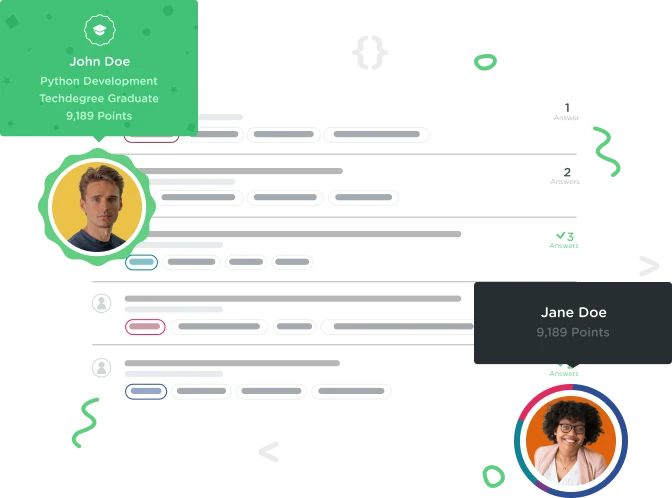

Unsubscribed User
96 PointsPlease help with this Java program
Program 1: Account.java 20 points
In this class, you will set up all of the components listed below. This class will not have a main method. It will be used to create objects in your MainApp class.
In your Account class:
Create 4 private data members A String that holds the account holder’s name An int that holds the checking account number An int that holds the user’s PIN number A double that holds the balance of the checking account Create 3 constructors A no-arg constructor where you set all data members with values of your choice A constructor that takes in 2 parameters (name and account number) and then sets the other two data members to values of your choice A constructor that takes in all 4 parameters and sets them Create a getter (accessor) and setter (modifier) for each data member. Use standard naming convention for these. Create a toString() method that displays the user’s account information (all 4 data members) in an easy-to-read format. (This isn’t realistic as far as the PIN number goes, but that’s okay.)
Make sure the standard requirements above compile before moving on.
Next, in your Account class:
Create an instance method called withdraw() that will take in a double and decrease the user’s account balance by the amount passed in. This will be a void method. You must include code inside this method that will prevent the user from setting their account balance to a negative value.
For example, if the account has $100 and the user attempts to withdraw $150, then the user method should decrease the balance from 100 to 0. You might add some print statements (this is okay) to let the user know that they can only withdraw $100.
Create an instance method called deposit() that will take in a double and increase the user’s account balance by the amount passed in. This will be a void method as well as we are just updating the balance. No checking mechanisms are required here. A confirmation print statement can be added if desired.
Make sure this compiles before moving on.