Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial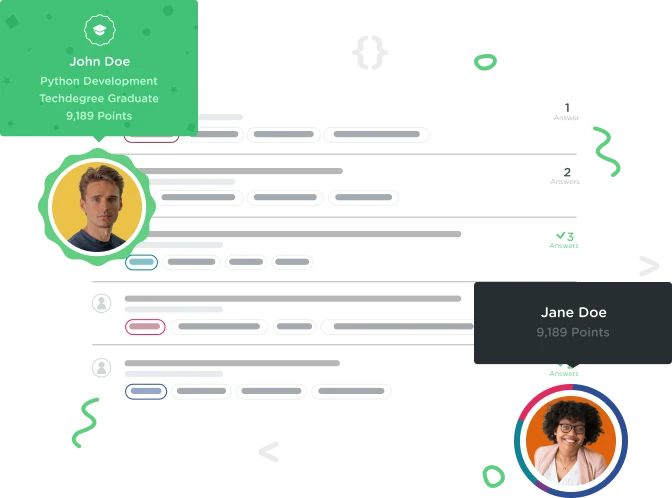

Amanda Lam
2,178 PointsPlease help me for the challenge!
Hi! I really need help for the challenge! I don't understand why my code won't work. The error I get is: "For category Food, expected 2 but received 3."
Here is my code:
public Map<String, Integer> getCategoryCounts() {
// putting all categories in a TreeSet
Set<String> categories = new TreeSet<String>();
for (BlogPost post: mPosts) {
categories.add(post.getCategory());
}
// counting categories
Map<String, Integer> countCategories = new HashMap<String, Integer>();
Integer count = 0;
for (String category : categories) {
for (BlogPost post: getPosts()) {
if (category == post.getCategory()) {
count++;
}
countCategories.put(category, count);
}
}
return countCategories;
}
1 Answer
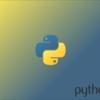
Kevin Faust
15,353 PointsHey Amanda,
You made the code alot more complicated that it had to be.
Ill break down how you were supposed to complete it.
So first thing, we can create a map inside our method. Creating a set was not necessary and made your code messy.
Map<String, Integer> map = new HashMap<>();
Next thing we want to do is loop through mPosts and do the following:
(1) the blogpost that we are currently looping on, we want to grab its category and store it as a variable so we can easily refer to it.
for (BlogPost bp : mPosts) {
String category = bp.getCategory();
}
What we want to do next is check if our map contains that category. we know that if we dont have the category, we want to add it and if we already have it, then we want to increase the count by 1. We use the following method on our map which will return a null value if we do not have it.
Integer count = map.get(category);
If we dont have the category, then we will initialize the count variable to 0
if (count == null) {
count=0;
}
so now, if we didnt have the category, our count would be 0. if we did have the category, the map.get()
method above wouldve stored how many counts of that category we had. we now just increase the count and put the category and the count back in the map. then return it
count++;
map.put(category, count);
}
return map;
}
I hope it made some sense to you. I posted the complete code below for your reference
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> map = new HashMap<>();
for (BlogPost bp : mPosts) {
String category = bp.getCategory();
Integer count = map.get(category);
if (count == null) {
count=0;
}
count++;
map.put(category, count);
}
return map;
}
Happy coding and best of luck,
Kevin
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsPS: In the future, use three black ticks (```) in front and at the end of your code so we can more easily see your code. You can also follow the first three black ticks by the language, in this case Java, for syntax highlighting. For more markdown tricks, click the "Markdown Cheatsheet" link below the comment box.
Mod edit: Modified comment to better explain markdown.
Amanda Lam
2,178 PointsAmanda Lam
2,178 PointsThanks so much. It finally works! :) Also, regarding the formatting - I did put the three black marks but I didn't know I was suppose to put java after it. But now I know!
Lee Reynolds Jr.
5,160 PointsLee Reynolds Jr.
5,160 PointsThis helped to clarify so much. You don't know how much I needed this. Thank you so much Kevin for breaking down the answer. It not only helped me get through the challenge, but, most importantly, it allowed me to see exactly where I was misunderstanding and go correct my understanding. I can't thank you enough.
Happy Coding :)
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsGlad to hear that
Happy coding to you too!