Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial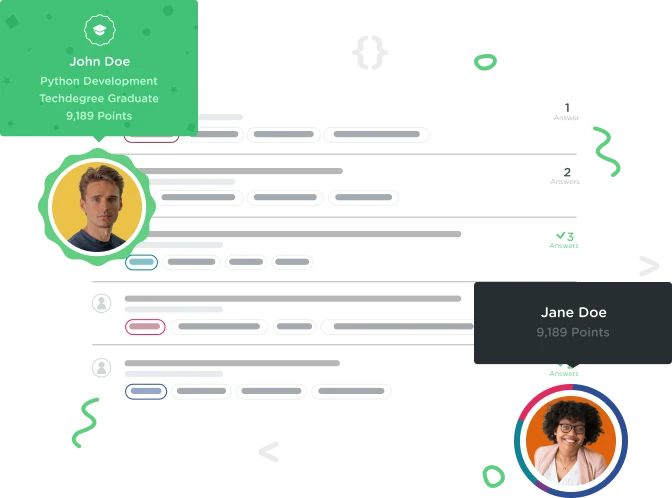

Andre Robinson
Courses Plus Student 6,057 PointsPlease help
I Think I'm having syntax error or I'm not assigning the properties right place help
#import <Foundation/Foundation.h>
@interface Treehouse : NSObject
@property (strong, nonatomic) NSDictionary *friends;
@end
#import "Treehouse.h"
@implementation Treehouse
- (instancetype)init
{
self = [super init];
if (self) {
_friends = @{@"firstName": @"Susan",
@"lastName": @"Olson",
@"profilePicture": @"susan_profile.png"
};
}
return self;
}
@end
#import <Foundation/Foundation.h>
@interface User : NSObject
@property (nonatomic, strong) NSString *firstName;
@property (nonatomic, strong) NSString *lastName;
@property (nonatomic, strong) NSString *profileImageName;
@property (nonatomic, strong) UIImage * profileImage;
@property (nonatomic, strong) UIImageView *userProfilePhoto;
@end
#import "User.h"
#import "Treehouse.h"
@implementation User
- (instancetype)init
{
self = [super init];
if (self) {
Treehouse *treehouse = [[Treehouse alloc] init];
NSDictionary *friendsDict = treehouse.friends;
_firstName = [friendsDict objectForKey:@"firstName"];
_lastName = [friendsDict objectForKey:@"lastName"];
//Enter your code below!
NSString * profileImageName = [friendsDictionary objectForKey:profilePicture];
friendsProfilePicture = [UIImage imageNamed:profileImageName];
return self;
}
return self;
}
@end
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey there!
What you are doing works, but you are not assigning the image nor the name to the properties defined in the header. I have added the corresponding task as comments to my answer.
#import "User.h"
#import "Treehouse.h"
@implementation User
- (instancetype)init
{
self = [super init];
if (self) {
Treehouse *treehouse = [[Treehouse alloc] init];
NSDictionary *friendsDict = treehouse.friends;
_firstName = [friendsDict objectForKey:@"firstName"];
_lastName = [friendsDict objectForKey:@"lastName"];
// Letβs start by retrieving the image name as a string from the friendsDict
// dictionary and storing that in the profileImageName property of the User
// model. In User.m, use the key profilePicture to get the relevant information.
_profileImageName = [friendsDict objectForKey:@"profilePicture"];
// Now that we have the image name as a string, letβs create a UIImage instance
// using the profileImageName property to retrieve an image. Store this UIImage
// instance in the profileImage property of the User model.
_profileImage = [UIImage imageNamed:_profileImageName];
// For our final task, we need to display the image we just created and to do
// that weβre going to use a UIImageView instance.
_userProfilePhoto = [[UIImageView alloc] initWithImage:_profileImage];
}
return self;
}
@end
Hope that helps :)