Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial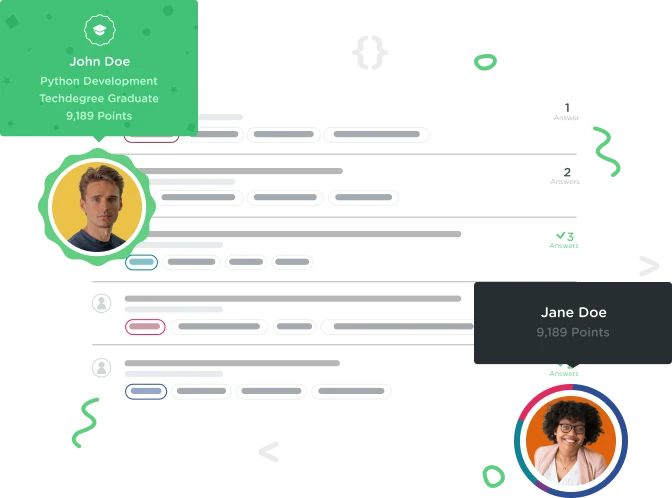
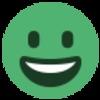
Jack Cummins
17,417 PointsPlease help.
Assignment: Can you please help me with the functions in the QuickFix.java over the next few tasks? I've included other code files for your reference only. I'm going to pass in this course to the addForgottenVideo method. For this task, can you please follow the TODOs in the comments in the method?
Thank You So Much! Jack
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
List<Video> = videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
videoList.add(1, video);
public void addForgottenVideo(Course course) {
List<Video> videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
videoList.add(1, video);
}
// TODO(2): Add the newly created video to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
return null;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Jack
I must say you need to modify your code a lot unfortunately. Especially in this area:
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
List<Video> = videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
videoList.add(1, video);
public void addForgottenVideo(Course course) {
List<Video> videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
videoList.add(1, video);
}
You got the principle quite alright when you create new Video object called video. Moreover you do fine when recognizing that you can call a List containing Video objects using course.getVideos() since the challenge already pass the Course type object called course in addForgottenVideo method.
However, you make new method exactly the same as the addForgottenVideo and let me remind you this is not polymorphism since the variable passed to the second addForgottenVideo is exactly the same as previous method which is a Course object called course. Thus you do not need to do this.
You need to keep everything simple that is the best advice I can give you right now. Just make new Video object called video. Then add it to List that you can directly access using course.getVideos() since if you check it will return a List of Videos and Course object has been passed to the addForgottenVideo method thus you can access it directly.
This is how I will code the addForgottenVideo method as simple as possible to tackle the first task of the challenge:
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
Video video = new Video("the Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
course.getVideos().add(1, video);
}
I hope this can help a little. Happy coding.