Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial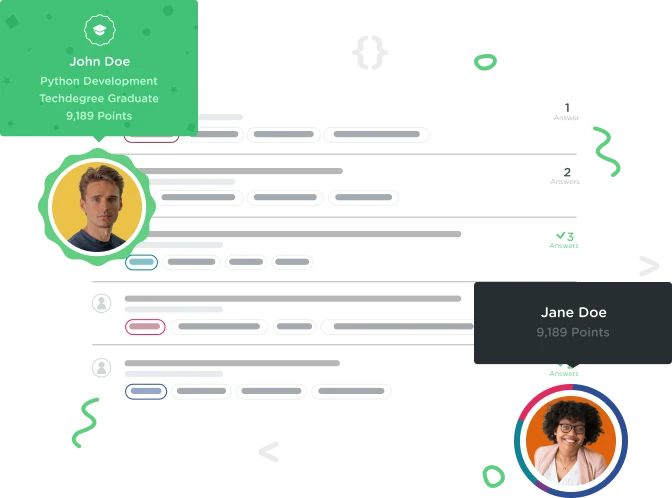

Zack Ball
4,958 PointsParse error: syntax error, unexpected end of file in /home/treehouse/workspace/inc/functions.php on line 206
Running into this error and I've tried numerous things.
functions.php
<?php
function get_catalog_count($category = null) {
$category = strtolower($category);
include("connection.php");
try {
$sql = "SELECT COUNT(media_id) FROM Media";
if (!empty($category)) {
$result = $db->prepare(
$sql
. " WHERE LOWER(category) = ?"
);
$result->bindParam(1,$category,PDO::PARAM_STR);
} else {
$result = $db->prepare($sql);
}
$result->execute();
} catch (Exception $e) {
echo "bad query";
}
$count = $result->fetchColumn(0);
return $count;
}
function full_catalog_array($limit = null, $offset = 0) {
include("connection.php");
try {
$sql = "SELECT media_id, title, category,img
FROM Media
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ',
''
),
'A ',
''
)";
if (is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1,$limit,PDO::PARAM_INT);
$results->bindParam(2,$offset,PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
}
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieved results";
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
function category_catalog_array($category, $limit = null, $offset = 0) {
include("connection.php");
$category = strtolower($category);
try {
$sql = "SELECT media_id, title, category,img
FROM Media
WHERE LOWER(category) = ?
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ',
''
),
'A ',
''
)";
if (is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1,$category,PDO::PARAM_STR);
$results->bindParam(2,$limit,PDO::PARAM_INT);
$results->bindParam(3,$offset,PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
$results->bindParam(1,$category,PDO::PARAM_STR);
}
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieved results";
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
function random_catalog_array() {
include("connection.php");
try {
$results = $db->query(
"SELECT media_id, title, category,img
FROM Media
ORDER BY RANDOM()
LIMIT 4"
);
} catch (Exception $e) {
echo "Unable to retrieved results";
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
function single_item_array($id) {
include("connection.php");
try {
$results = $db->prepare(
"SELECT title, category, img, format, year,
publisher, isbn, genre
FROM Media
JOIN Genres ON Media.genre_id=Genres.genre_id
LEFT OUTER JOIN Books
ON Media.media_id = Books.media_id
WHERE Media.media_id = ?"
);
$results->bindParam(1,$id,PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "bad query";
echo $e;
}
$item = $results->fetch(PDO::FETCH_ASSOC);
try {
$result = $db->prepare("
SELECT fullname,role
FROM Media_People
JOIN People ON Media_People.people_id=People.people_id
WHERE media_id = ?");
$result->bindParam(1,$id,PDO::PARAM_INT);
$result->execute();
} catch (Exception $e) {
echo "bad query";
echo $e;
}
while ($row = $result->fetch(PDO::FETCH_ASSOC)) {
$item[$row["role"]][] = $row["fullname"];
}
return $item;
}
function genre_array($category = null) {
$category =strtolower($category);
include("connection.php");
try {
$sql = "SELECT genre, category"
. " FROM Genres "
. " JOIN Genre_Categories "
. " ON Genres.genre_id = Genre_Categories.genre_id ";
if (!empty($category)) {
$results = $db->prepare($sql . " ORDER BY genre"
. " WHERE LOWER($category) = ?"
. " ORDER BY genre");
$results->bindParam(1,$cateogry,PDO::PARAM_STR);
} else {
$results = $db->prepare($sql . " ORDER BY genre");
}
$results->execute();
} catch (Exception $e) {
echo "bad query";
}
$genres = array();
while ($row = $results->fetch(PDO::FETCH_ASSOC)) {
$genres[$row["category"]][] = $row["grenre"];
}
return $genres;
function get_item_html($item) {
$output = "<li><a href='details.php?id="
. $item["media_id"] . "'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
function array_category($catalog,$category) {
$output = array();
foreach ($catalog as $id => $item) {
if ($category == null OR strtolower($category) == strtolower($item["category"])) {
$sort = $item["title"];
$sort = ltrim($sort,"The ");
$sort = ltrim($sort,"A ");
$sort = ltrim($sort,"An ");
$output[$id] = $sort;
}
}
asort($output);
return array_keys($output);
}
?>
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Zach,
It looks like your function genre_array
doesn't have a closing curly brace.
Vasil Dajko
3,018 PointsVasil Dajko
3,018 PointsI guess you should check you opening and closing tags { } you might have missed one