Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial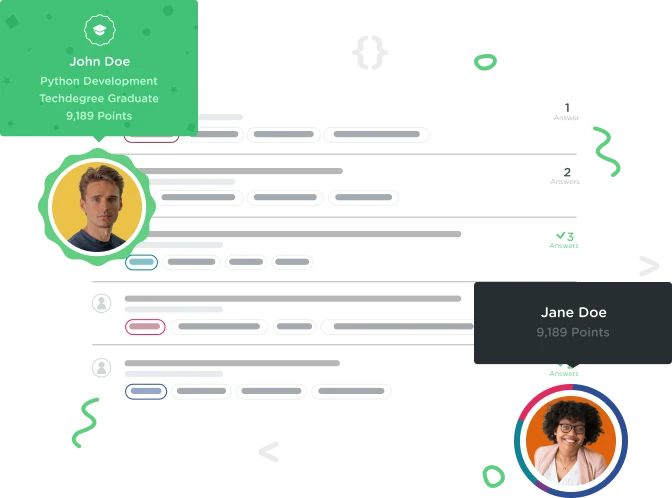
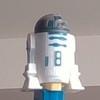
Teacher Russell
16,873 Pointsorganizing your code with require
I think I understand what's going on, but I can't understand the explanation of why get and not get profile. Andrew says use module.exports, then the name of our API, get, then set it to something. "In this case, the get function." So, both are set to the get function?
It seems that if you kept the name of the function getProfile, it would just be module.exports.getProfile = get. What's wrong with that, and why does it have to be set to get in the first place?
I've watched this a dozen or so times. I'm sorry, it's just not at all clear.
Can someone explain what is actually going on here? Thanks all!
4 Answers
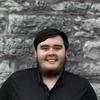
Michael Hulet
47,913 Pointsmodule.exports
is an object containing everything another file will be able to see/use when it require
s your module. Ultimately, you could name your functions and variables whatever you want, both inside and outside your module. This:
module.exports.getProfile = get;
is just as valid as this:
module.exports.get = get;
The only difference would be instead of referencing that function like this:
const profile = require("./profile.js");
profile.get();
You'd have to reference it like this:
const profile = require("./profile.js");
profile.getProfile();
While it's not required, JavaScript API designers often just kinda assume that the users of their module are going to store it in a variable named exactly how they named their module (though it isn't technically required). So, if you store your profile.js
module in a variable called profile
, you already have the information visually of what you're getting, so the convention is that naming it like profile.getProfile
is redundant, so many developers choose to just name it profile.get
instead. However, you can name it whatever you'd like and it'd still work fine
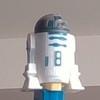
Teacher Russell
16,873 PointsGot it! Except that, if we had left the function appropriately named getProfile, the only difference is this. module.exports.get = getProfile; In app.js, it's still users.forEach(profile.get); It's not redundant, and the function better describes what it's doing. Changing that function name seems pretty pointless and unnecessarily confusing, being that they have the same name. The name change of the function is the only thing that had me thinking I was confused. Thanks again!
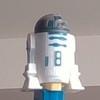
Teacher Russell
16,873 PointsI feel I get all of that. I still don't understand what he means when he says that if we named the function getProfile, the API we created would just be get. It seems to fly in the face of everything else he says, and what I see with my own eyes. I feel like I understand your explanation, and his, except that it doesn't explain what he means when he says "WOULD be just get". It IS named get. The function is named get, the name given to it is get. Sorry, I guess I'm a bit thick. I can't see the connection between that sentence and any other part of the explanation. I am a native English speaker, for the record, but perhaps I'm just misunderstanding what he's saying there. Again, in short, I'm hearing ...if we named it getProfile, it would be named get.
Thanks a lot for your time. I appreciate the help and all of the effort. If not for that one sentence, I'd believe I understand all of this completely.
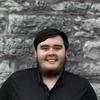
Michael Hulet
47,913 PointsSay that inside of profile.js
, we declared the function like this:
function getProfile(){
// Code here...
}
module.exports.get = getProfile;
What he's saying is that inside of the profile.js
file, you'd call the function like this:
getProfile();
Anywhere else that require
s profile.js
, you'd call it like this:
const profile = require("./profile.js");
profile.get();
Inside of profile.js
, it'd be called getProfile
, but outside of profile.js
, it'd be get
. That's in contrast to what is ultimately shown in the video, where it's get
both inside and outside of profile.js
. That's what he's trying to say there
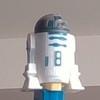
Teacher Russell
16,873 PointsThanks again. All that work, I have to give you best answer, and I'm sure it's there somewhere:) From the video, I had the idea that the first .get is referring to the name of the function, the second get being an ID of sorts. In other words, originally it would have gone module.exports.getProfile = get; It seems that isn't the case. I can't get any other variation that makes sense to me to work. In summation, I'm much more confused than I had realized. I'm going to hit the docs and youtube and see if I can make some sense out of this. Thank you, sir.
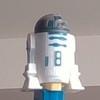
Teacher Russell
16,873 PointsI can't this to work as getProfile() module.exports.getProfile = get; The 2 must match. The function must match what follows module.exports , which must equal itself. I've tried every variation.
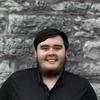
Michael Hulet
47,913 Pointsmodule.exports.getProfile = get;
is perfectly valid, as long as the function is declared like this inside of profile.js
:
function get(){
// Code here...
}
module.exports.getProfile = get;
Inside of profile.js
, you'd call the function like get();
, but in any other file, you'd call it like this:
const profile = require("./profile.js");
profile.getProfile();
Teacher Russell
16,873 PointsTeacher Russell
16,873 PointsMakes sense to me, so why does he say that if we went back and named our function getProfile, our API would just be named get? That's the sentence that baffles me:) It IS just named get. What does he mean by that?
Michael Hulet
47,913 PointsMichael Hulet
47,913 PointsHe's saying that the fact that the function is named
get
insideprofile.js
and the name of the exported function is alsoget
is just a coincidence, and they can be named differently, if you so choose. For example:In this case, the name of the function inside of
profile.js
isget
, and if you want to call/reference it inside ofprofile.js
, you have to call it like this:get();
. If you call it asgetProfile();
, it willTypeError
. However, its exported name isgetProfile
, so in order to call that function in another file, you have to call it asprofile.getProfile();
, as callingprofile.get();
willTypeError
because that file can't see a function namedget
.