Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial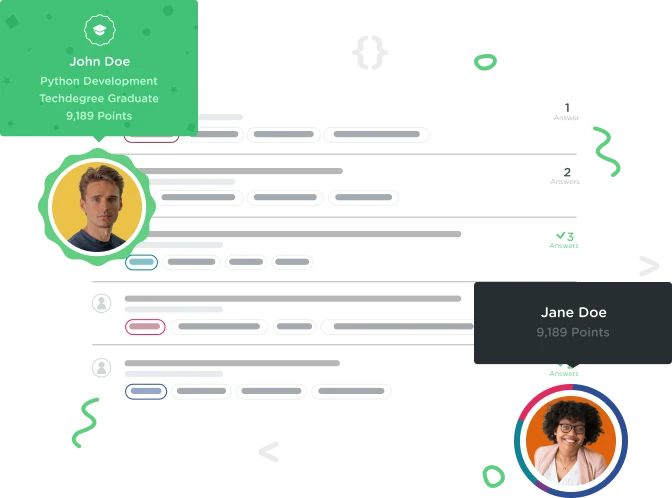
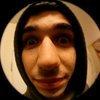
Thomas Marren
3,626 PointsOnly one question shows up on my list
When I run this code the only question that shows up on the webpage is "What is the capital of the USA?" If I answer it correct it goes in the correct array if I answer it incorrect it goes in the incorrect array and the other array says undefined on the webpage.
What am I doing wrong?
function print(message) {
document.write(message);
}
var questions = [
['What is the capital of NY?' , 'Albany'] ,
['What is the capital of PA?' , 'Harrisburg'] ,
['What is the capital of the USA?' , 'Washington DC']
];
var score = 0;
var correct = [ ];
var incorrect = [ ];
//ask the questions
for (var i = 0; i < questions.length; i++) {
var userAnswer = prompt(questions[i][0]);
if ( userAnswer === questions[i][1] ) {
correct.push(questions[i][0]);
score += 1;
} else {
incorrect.push(questions[i][0]);
}
}
//print the total number of questions correct
print('You got ' + score + ' question(s) right');
//create a list of each array for correct and incorrect
for (var i = 0; i < correct.length; i++){
var correctList = '<ol>';
correctList += '<li>' + correct[i] + '</li>';
correctList += '</ol>';
}
for (var i = 0; i < incorrect.length; i++){
var incorrectList = '<ol>';
incorrectList += '<li>' + incorrect[i] + '</li>';
incorrectList += '</ol>';
}
print("You got these questions right " + correctList);
print("You got these questions wrong " + incorrectList);
1 Answer
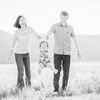
Shawn Boyd
14,345 PointsIn your loops for printing out the list of answered and unanswered questions you are initializing the variable holding the ordered list with each iteration of the loop. Meaning that only the last iteration, if there is one, will be held within the variable. If one of the for loops never runs because all of the answers were correct or all were incorrect then that variable (correctList or incorrectList) will never be initialized and result in an undefined value when you get to the print command.
To fix this, initialize the variable that you'll use to hold the list items outside of the for loop, including opening and closing ol tags. Inside the loop you should only be adding list items, as appropriate. Something like this...
...
//start ordered list
var correctList = '<ol>';
//add questions answered correctly, if any
for (var i = 0; i < correct.length; i++){
correctList += '<li>' + correct[i] + '</li>';
}
//close ordered list
correctList += '</ol>';
//start ordered list
var incorrectList = '<ol>';
//add questions answered incorrectly, if any
for (var i = 0; i < incorrect.length; i++){
incorrectList += '<li>' + incorrect[i] + '</li>';
}
//close ordered list
incorrectList += '</ol>';
//print list of correct and incorrect
print("You got these questions right " + correctList);
print("You got these questions wrong " + incorrectList);
Thomas Marren
3,626 PointsThomas Marren
3,626 PointsThanks Shawn! This was driving me crazy. I wound up scraping the whole thing and creating a function. But this definitely helped me understand to be careful with what goes into the for loops.