Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial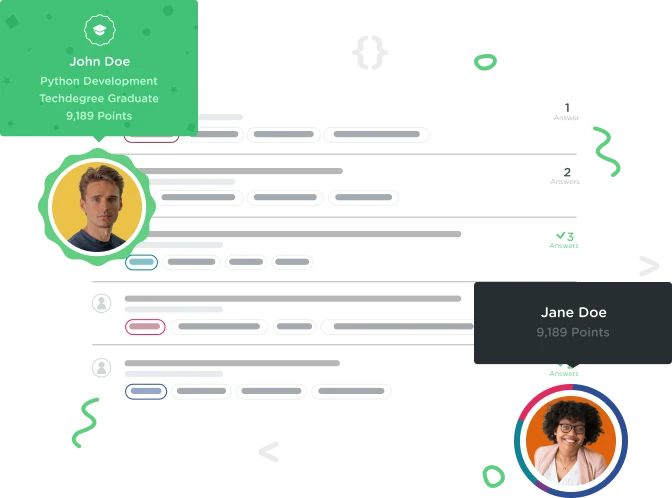
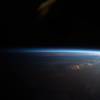
Jay G
2,904 PointsOn line 24, if I have 3 equals the conditional statement fails, but with 2 equals it passes. Would love to know why.
I understand that 3 equals is a strict comparison, but what am I am missing here? Just copied and pasted and see that the numbers don't come along with the code. So in my quiz function, once I prompt the user for their guess, the conditional statement that asks if guessAnswer == answers[i]. Thank you very much.
function print(message) {
document.write(message);
}
var questionArray = [
['How many states are there?'],
['What is the biggest number up to 10?'],
['What is better, a cat or dog?']
];
var answerArray = [
['50'],
['10'],
['dog']
];
var quiz = function(questions, answers){
var correctHTML = '<ol><h1>This is what you got right</h1>';
var incorrectHTML = '<ol><h1>This is what you got wrong</h1>';
var guessAnswer;
for(var i = 0; i < questions.length; i+= 1){
guessAnswer = prompt(questions[i]);
if(guessAnswer == answers[i]){
correctHTML += '<li>' + questions[i] + '</li>';
correctHTML += '<ul>' + answers[i] + '</ul>';
} else {
incorrectHTML += '<li>' + questions[i] + '</li>';
incorrectHTML += '<ul>The correct answer: ' + answers[i] + '</ul>';
incorrectHTML += '<ul>What you guessed: ' + guessAnswer + '</ul>';
}
}
correctHTML += '</ol>';
incorrectHTML += '</ol>';
print(correctHTML + '<p>' + incorrectHTML + '</>')
};
quiz(questionArray, answerArray);
2 Answers
Seth Shober
30,240 PointsHi Jason,
The issue lies in answerArray
. What I did to troubleshoot this was think, "Hmm, if there's an issue with triple equals the types must not be matching." I added some console.log()
statements:
console.log(typeof guessAnswer); // 'string'
console.log(typeof answers[i]); // 'object'
console.log(answers); // [Array[1], Array[1], Array[1]]
We would be expecting guessAnswer
and answers[i]
to both be strings. The output for answers
also looked a bit funky. Since answers seemed to be showing unexpected behavior I analyzed the answerArray
variable, which was the argument being passed as the answers parameter. What I noticed is that you defined each index in the array as another array, and in JavaScript arrays are of the type 'object'. So, what you actually have is an array of arrays, when what I think you would prefer is an array of strings.
I hope this provides the clarity you were looking for.
Cheers!
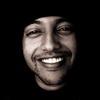
miikis
44,957 PointsHey Jason,
The problem is your answerArray β you put its values as arrays... so now you have a two dimensional array. This isn't necessary and when you use the ===, it evaluates to false. So change the values of your answerArray to just strings and === will work.
miikis
44,957 Pointsmiikis
44,957 PointsHa beat me to it seΓ±or. Good answer too.
Jay G
2,904 PointsJay G
2,904 PointsThank you for the thorough response. I totally forgot that I could throw a debugger in and see what is happening where the conditional statement was. Thanks again