Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial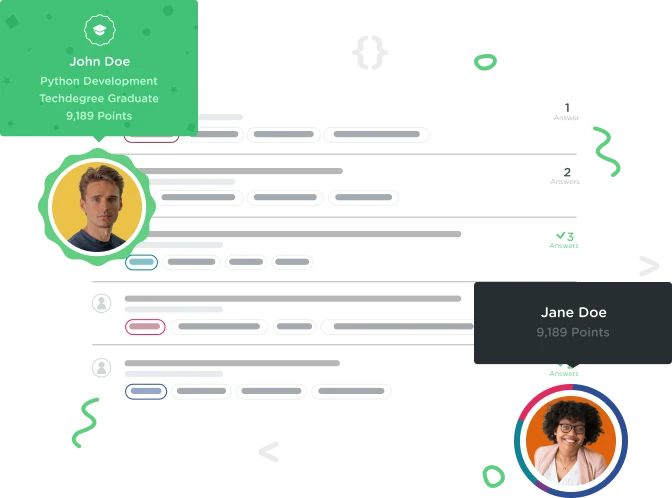

Abhilasha jangid
2,925 Pointsnow I did not understand what it ask
Make sure you've added a new constructor to the ForumPost class that accepts a User named author, a String named title, and another String named description
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic){
topic = "abhilasha";
}
public String getTopic() {
return topic;
}
/* Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
*/
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
firstName = "Abhilasha";
lastName ="Jangid";
}
// TODO: add getters for firstName and lastName
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public class User{
private String title;
private String description;
public User (String title,String description){
this.title = title("wcbic");
this.description =description("tukenth");
}
}
}
}
2 Answers
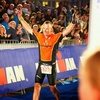
Steve Hunter
57,712 PointsHi again,
There are three constructors to write in this challenge. There's one in User
, another in Forum
and one more in ForumPost
.
All of these constructors take some parameters. These are variable that are passed into the method to set a member variable inside the class. None of these are hard coded using actual strings, names, topics or descriptions. Let's start with the Forum
constructor as it is the most simple.
We have this:
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
The class has one member variable called topic
. It is a String. The comment tells us that we want to set the value of this string from the constructor. That means the constructor will receive a string argument/parameter that we can use to do this. A constructor is usually public
and always called the same as the class; we know this one receives a string which we'll call topic
. Setting the member variable is done by using the this
keyword. Putting all that together gives us:
public class Forum {
private String topic;
public Forum(String topic){
this.topic = topic;
}
The same process can be adopted for all the other constructor. See what the constructor is expecting to set inside the class (the member variables), make sure the constructor receives something of that datatype (User
, String
) etc. And then set the member variables inside the constructor.
I hope that helps - post your code for each constructor in here and we can work through them.
Steve.

Abhilasha jangid
2,925 Pointspublic class User{
private String title;
private String description;
public User(String title, String description){
this.description= description;
this.title = title;
}
whats wrong here
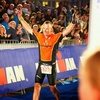
Steve Hunter
57,712 PointsHere, you've got mixed up between two classes.
This is the User
class. A User
has two member variables both of which are String
s. There's a firstName
and a lastName
.
What you've nearly created is the constructor for the ForumPost
. That has a title
, a description
(both strings) but also a User
called author
. So, to edit your code slightly gives us the constructor for the ForumPost
objects:
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description){
this.author = author;
this.title = title;
this.description = description;
}
// rest omitted
Make sense?
Steve.