Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial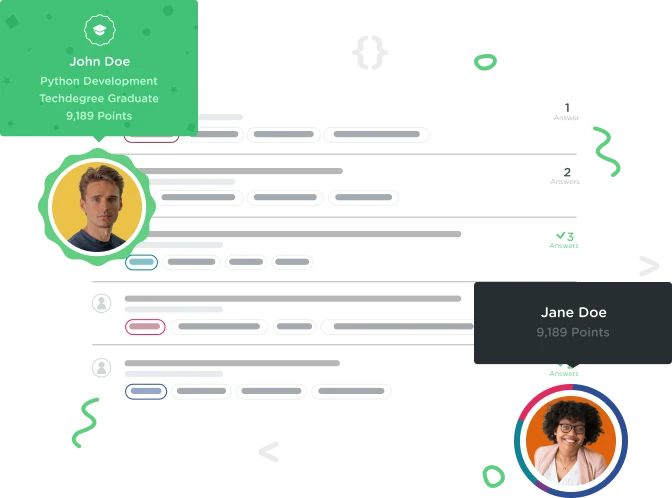

Mel Rojo
Full Stack JavaScript Techdegree Student 4,699 PointsNot sure how to solve this challenge
Does anyone know how to solve this challenge?
function reformatName(text) {
const rawName = /^(\w+)\s(\w+)$/;
// Type your answer on line 5, below:
return text.replace(rawName, newText);
}
const form = document.querySelector("form");
const input = form.querySelector("input");
const reformatted = document.getElementById("reformatted");
form.addEventListener("submit", e => {
e.preventDefault();
reformatted.textContent = reformatName(input.value);
});
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<form>
<label for="name">Enter your first and last name, please.</label>
<br />
<input type="text" id="name" name="name">
<button type="submit">Reformat</button>
</form>
<div>
<h2>Reformatted name:</h2>
<p id="reformatted"></p>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
3 Answers

Joyce van den Berg
10,684 PointsIt's a bit intimidating, but you really have to closely read the requirements. I could just give you the answer, but it would help most if you specifically said where you are stuck. I assume you are stuck at the very beginning. I know I first thought 'what??' when I saw this. So here goes the start.
What is being captured by the regex in the given const is a name: first and last. It is captured in 2 groups of letters/characters. You can tell this from the (\w+) which captures one group of characters before a space and then the last group of characters after the space. So far so good. 'All you need to do is' ... switch the groups around and add a comma between them in the new variable.
Create a variable newText, and assign a replacement string to that variable. So you need to remember how to call on a group and add that to a variable. Go to the video before, to about 3 minutes in. That should help you :)

Mel Rojo
Full Stack JavaScript Techdegree Student 4,699 PointsHi Joyce, thanks for your message.
I tried as you said to: "switch the groups around and add a comma between them in the new variable.".
function reformatName(text) {
const rawName = /^(\w+)\s(\w+)$/;
// Type your answer on line 5, below:
const newText = /(\w+)$[^,\s]^(\w+)/;
return text.replace(rawName, newText);
}
const form = document.querySelector("form");
const input = form.querySelector("input");
const reformatted = document.getElementById("reformatted");
form.addEventListener("submit", e => {
e.preventDefault();
reformatted.textContent = reformatName(input.value);
});
I went through the videos again but I still don't quite understand how this works....

Joyce van den Berg
10,684 PointsI think I see where the problem is. In the const rawName = /^(\w+)\s(\w+)$/; you capture a regular expression that locates first a couple of characters in the first group: (\w+). Then a space: s. And then another group of characters: (\w+). If you create a new variable with that regular expression you don't really do anything other than add a comma. And that is not what you need to do. You need to take the captured information (the two groups of characters) and use them in the new variable. But not as a regular expression. In the video around 3 minutes in there is an explanation of how you access those groups the regex found/captured.
It's something with a number to find the first group and then the second. That way you can indicate which group needs to be presented first and which one needs to be presented second. Because the input is first name-space-second name, right? But you need to replace that with SECOND name, first name. That needs to go in the new variable.
I'll think of a way to be more precise without just giving you the answer, because I'd like for you to understand it and not just pass the quiz :)