Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial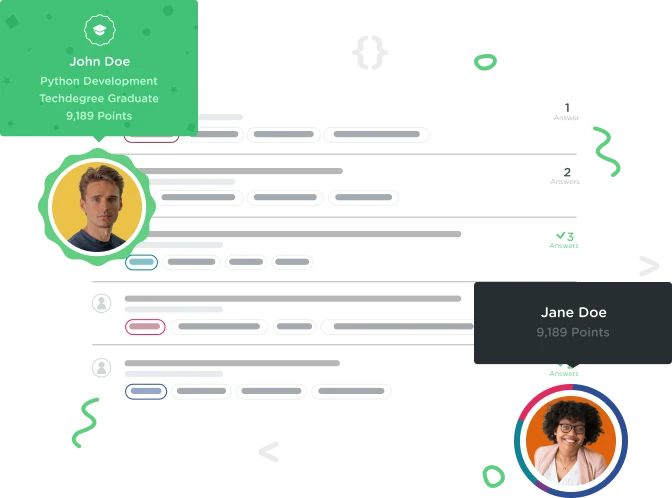
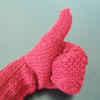
Jonathan Mitten
Courses Plus Student 11,197 PointsNormalizing Discount Code results in Task 1 no longer working.
As far as I can tell, I'm executing this properly. Task 1 was of course working before the introduction to the dollar sign. The ability to debug being limited, I get the code to run but the result is "Oops! It looks like Task 1 is no longer working".
Can anybody spot a flaw here?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
discountCode = discountCode.toUpperCase();
for (char letter: discountCode.toCharArray()){
if ( ! Character.isLetter(letter) || letter != '$' ){
throw new IllegalArgumentException("Invalid discount code.");
}
}
return discountCode;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers

Alex Bratkovskij
5,329 PointsHeya Jonathan
All is good with your code, there is a small mishap though, your not sign(!) should be either right next tot the Character.isLetter(), or just encapsulate the whole statement and make it not letter nor a dollar sign and all will work like a charm
private String normalizeDiscountCode(String discountCode){
discountCode = discountCode.toUpperCase();
for (char letter : discountCode.toCharArray()){
if ( ! Character.isLetter(letter) || letter != '$' ){ // your not sign (!) should enclose both statements
throw new IllegalArgumentException("Invalid discount code.");
}
}
return discountCode;
}
Good luck !
-Alex

andren
28,558 PointsThere is a flaw in your code, but it's a very common flaw for this task. Probably because of how the task is phrased.
To illustrate the flaw I'll walk through what will happen with your application when a discount code is passed in. Let's say the discount code is F$L
which is a valid code and should not throw an error.
First your code will check if F
is not a letter, since it is a letter this will be false, then it will check if it is not $
. Since F
is in fact not $
this condition will be true. Since you use the OR (||) operator your if statement will run as long as one of the conditions is true. F
will therefore cause the if statement to run which will trigger the exception. Even though F
is in fact a valid character. The same sort of error occurs with $
except that the first condition is true while the second is false.
There are multiple ways of avoiding this issue but the simplest is probably to change the || operator to &&. && will only return true if both of the conditions are true, which is the behavior you actually want from the if statement you have written.