Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial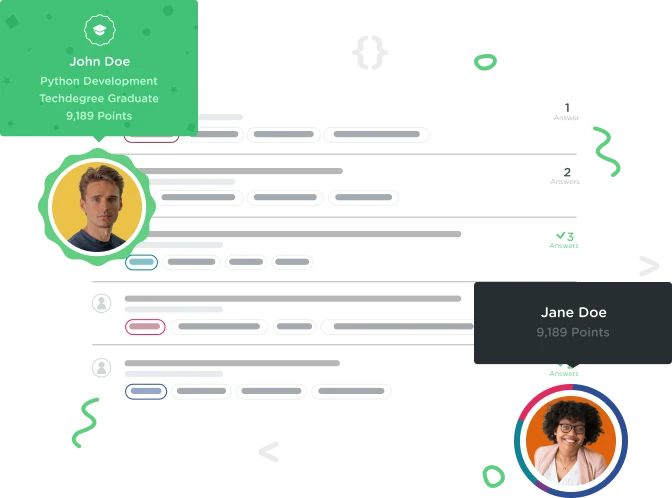

Chris Thomas
Front End Web Development Techdegree Graduate 34,909 PointsNested Array Iteration Code Challenge
I understand the concept that the question is asking but having trouble walking through it. Is it possible just calling the .reduce() method once or will I have to use other methods?
Since the object I need to access is nested within another object, should I create an array of those secondary objects and then "flatten" the array with .reduce()?
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers.reduce();
3 Answers
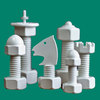
Steven Parker
241,958 PointsIt's possible to solve the challenge with a single call to "reduce".
If you were able to do that with the previous challenge, this one should only be slightly different.

Chris Thomas
Front End Web Development Techdegree Graduate 34,909 PointsOkay that's what I thought. I'm just mixing a few things up I believe. I'm trying to follow MDN's documentation on flattening arrays of arrays.
hobbies = customers.reduce((personal, hobby) => personal.concat(hobby), []);
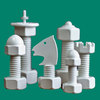
Steven Parker
241,958 PointsThat looks like a solution for the last one. For this one, you'll need to access the right property from each array item.

Iain Simmons
Treehouse Moderator 32,305 PointsThe main thing to keep in mind are what the first two arguments for the callback function will be in each iteration.
The first is the accumulator, which is essentially the empty array that you start with, and then the updated array as you add each hobby.
The second is the currentValue, which in this case will be an object for each customer.
And you want to return an updated array/list. So you can use the concat
method, but you need to access the nested array. Hint: use dot notation to get the hobbies
array.