Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial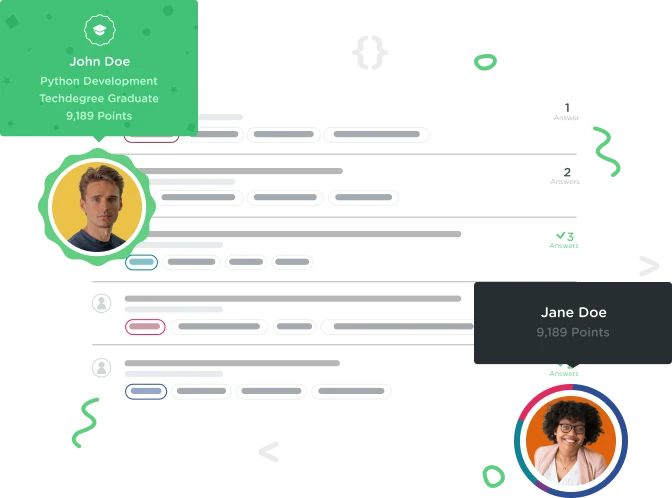

Ronnie Glickman
5,866 PointsNeed help with the challenge below please!
In the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used, throw a IllegalArgumentException with the message Invalid discount code.
I have been unable to figure out how to apply the skills I learned in the video to complete this challenge. I thought if statement would be best way to tackle it but am running into the issue of checking for the $ symbol. Did some research and tried using the regex method to write the code and still seemed to find errors with it. Can anyone please help by writing out the long and short way to best write this code? Thanks!
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
return discountCode.toUpperCase();
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
3 Answers
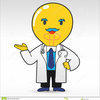
Cameron Hastings
3,439 PointsHere is what worked for me:
private String normalizeDiscountCode(String discountCode) {
for (int i = 0; i < discountCode.length(); i++) {
char [] ndc = discountCode.toCharArray();
if (! Character.isLetter(ndc[i]) && ndc[i] != '$') {
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
I used this post from 21 days ago to help me out.
You need to use a 'for' loop which was used in the video. In the 'for' loop, it sets 'i = 0' and gets the number of characters used in 'discountCode' by using 'discountCode.length().' It then adds 1 to 'i' every time it goes through the loop. The loop continues as long as 'i < discountCode.length();'
The 'char [] ndc = discountCode.toCharArray();' creates an array of every letter, number, and symbol in discountCode.
It then goes though an if statement for every character in the ndc array. If it is not a letter (! Character.isLetter(ndc[i]) and if it is not equal to '$', then 'throw new IllegalArgument Exception("Invalid discount code");
It then returns 'discountCode.toUppercase()' like you did in step 1.

nazim khan
1,409 PointsThanks Cameron... That worked for me also but I thing we can even reduce the code using for each loop
private String normalizeDiscountCode(String discountCode) {
for (char c:discountCode.toCharArray())
{
if (! Character.isLetter(c) && c != '$')
{
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}

Thandolwethu Bhebhe
1,417 Pointsbut arent you you checking if your character is not equal to $ in your if statement? dont we want the $?

Brice Roberts
22,415 PointsYes, this code is checking for non-equality, because we need to return the InvalidArgumentException if the characters are not equal to a valid letter or "&".
If the characters are equal to a valid letter or "&" we do not need an error.
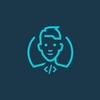
Luka Sarich
13,204 PointsWhat is the 'c' in the for loop declaration?
for (char c:discountCode.toCharArray())

Brice Roberts
22,415 PointsIt is a declared variable for the For Loop. It is not setting it to the actual character c, c is just the name of the variable. c could be anything. c here is just shorthand for the word character.
It could just as easily be..
for (char placeholder:discountCode.toCharArray())
We are looking for a character named c, inside the string "discountCode" which we are turning into an array of characters.
Then, in the IF statement, you are checking if c is a letter or is not equal to "$".
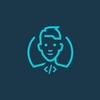
Luka Sarich
13,204 PointsThank you for the thorough explanation! That is what I was assuming, but I just wanted to be sure. Thanks again.
Carlos Salcedo
1,788 PointsCarlos Salcedo
1,788 Pointsgreat explanation. helped me out alot i was really stuck. thanks alot.
chase singhofen
3,811 Pointschase singhofen
3,811 Pointsworked good. i had no idea how to use the for loop in this one. i new i needed an exception as well. didnt even think i should put the
public void applyDiscountCode(String discountCode) { this.discountCode = normalizeDiscountCode(discountCode); }
at the bottom